


How Can I Effectively Manage Global Variables in Multi-File Projects?
Implementing Global Variables in Multi-File Projects
Managing global variables across multiple files can be a perplexing task. This article will guide you through two common approaches to declare shared variables that can be accessed consistently throughout your project.
Method 1: Using a Settings File
This approach involves creating a separate file, typically named "settings.py," responsible for defining and initializing global variables. The settings file should contain an "init()" function that initializes the variables:
# settings.py def init(): global myList myList = []
Other files in your project can then import the "settings.py" file and use the global variables:
# other_file.py import settings def stuff(): settings.myList.append('hey')
Method 2: Importing a Module with Global Variables
An alternative method is to create a module that defines the global variables and import that module into the files that require access:
# my_globals.py global myList myList = []
Other files can then import the "my_globals.py" module and access the global variable:
# other_file.py import my_globals def stuff(): my_globals.myList.append('hey')
Initialization and Reuse
It's important to note that the "init()" function in the "settings.py" file should be called only once in the main module (e.g., "main.py"). This ensures that global variables are initialized correctly and avoids potential errors caused by multiple initialization attempts.
By following these approaches, you can effectively manage global variables across multiple files in your project, ensuring consistent data sharing and facilitating efficient code organization.
The above is the detailed content of How Can I Effectively Manage Global Variables in Multi-File Projects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


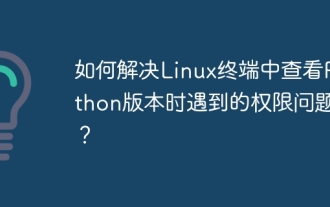
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
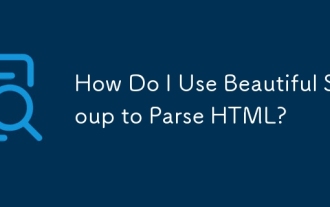
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
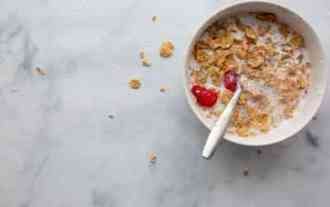
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
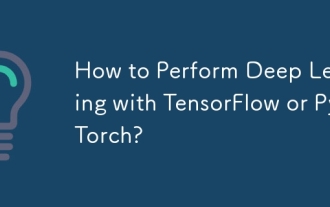
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
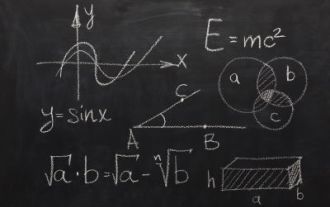
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti

This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
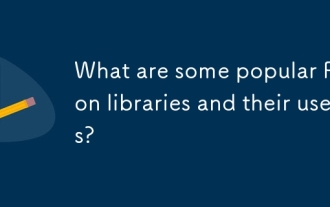
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
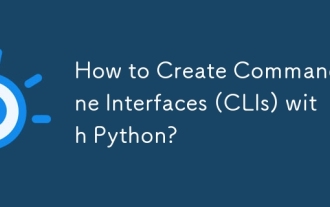
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
