


How to Properly Retrieve MySQL Data Using jQuery AJAX and Address Deprecated PHP Functions?
Using jQuery AJAX to Retrieve Data from MySQL
Retrieving data from a MySQL database using jQuery AJAX is a common task in web development. However, there may be instances where the code fails to work as intended.
One such instance is when attempting to display records from a MySQL table via an Ajax call. The provided code snippet:
Records.php: <?php //database name = "simple_ajax" //table name = "users" $con = mysql_connect("localhost","root",""); $dbs = mysql_select_db("simple_ajax",$con); $result= mysql_query("select * from users"); $array = mysql_fetch_row($result); ?>
and
list.php: <html> <head> <script src="jquery-1.9.1.min.js"> <script> $(document).ready(function() { var response = ''; $.ajax({ type: "GET", url: "Records.php", async: false, success: function(text) { response = text; } }); alert(response); }); </script> </head> <body> <div>
is not functioning as expected. The issue may lie in the use of deprecated PHP functions. To resolve this, the code should be updated to use mysqli_connect instead of mysql_connect, mysqli_select_db instead of mysql_select_db, and mysqli_query instead of mysql_query.
Furthermore, for retrieving data using Ajax jQuery, the following code snippet can be used:
<html> <script type="text/javascript" src="jquery-1.3.2.js"> </script> <script type="text/javascript"> $(document).ready(function() { $("#display").click(function() { $.ajax({ //create an ajax request to display.php type: "GET", url: "display.php", dataType: "html", //expect html to be returned success: function(response){ $("#responsecontainer").html(response); //alert(response); } }); }); }); </script> <body> <h3>Manage Student Details</h3> <table border="1" align="center"> <tr> <td> <input type="button">
For MySQLi connection, use the following code:
<?php $con=mysqli_connect("localhost","root",""); ?>
To display data from the database:
<?php include("connection.php"); mysqli_select_db("samples",$con); $result=mysqli_query("select * from student",$con); echo "<table border='1' > <tr'> <td align=center> <b>Roll No</b></td> <td align=center><b>Name</b></td> <td align=center><b>Address</b></td> <td align=center><b>Stream</b></td> <td align=center><b>Status</b></td>"; while($data = mysqli_fetch_row($result)) { echo "<tr>"; echo "<td align=center>$data[0]</td>"; echo "<td align=center>$data[1]</td>"; echo "<td align=center>$data[2]</td>"; echo "<td align=center>$data[3]</td>"; echo "<td align=center>$data[4]</td>"; echo "</tr>"; } echo "</table>"; ?>
The above is the detailed content of How to Properly Retrieve MySQL Data Using jQuery AJAX and Address Deprecated PHP Functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
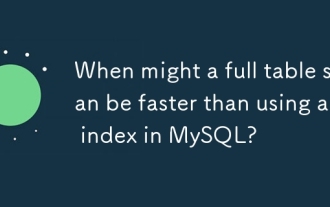
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
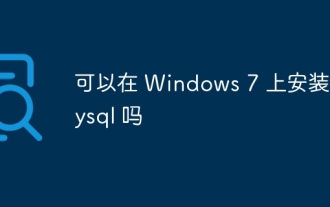
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
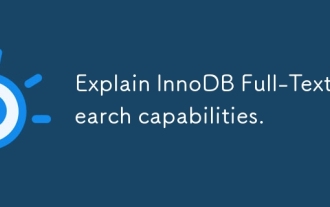
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
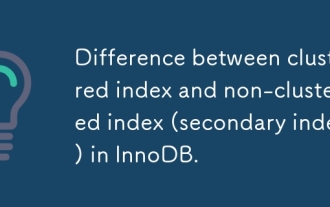
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
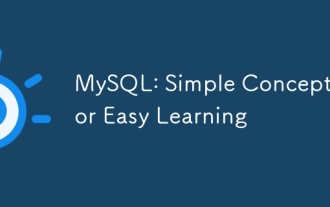
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
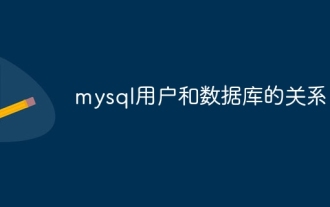
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
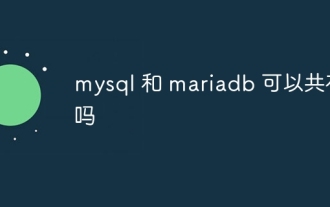
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
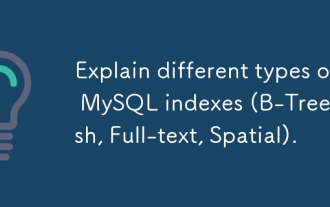
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
