


How to Generate Java Classes from JSON Using the jsonschema2pojo Maven Plugin?
Dec 09, 2024 am 04:45 AMHow to Generate Java Classes from JSON in Maven
Generating Java source files from JSON is a valuable technique for object-to-JSON mapping and data serialization. In this scenario, we seek to create Java classes resembling the following:
class Address { private JSONObject mInternalJSONObject; Address(JSONObject json) { mInternalJSONObject = json; } String getStreetAddress() { return mInternalJSONObject.getString("streetAddress"); } String getCity() { return mInternalJSONObject.getString("city"); } } class Person { private JSONObject mInternalJSONObject; Person(JSONObject json) { mInternalJSONObject = json; } String getFirstName() { return mInternalJSONObject.getString("firstName"); } String getLastName() { return mInternalJSONObject.getString("lastName"); } Address getAddress() { return new Address(mInternalJSONObject.getJSONObject("address")); } }
To achieve this generation in a Maven project, you can leverage a comprehensive tool such as http://www.jsonschema2pojo.org. Alternatively, you can utilize the jsonschema2pojo plug-in for Maven:
<plugin> <groupId>org.jsonschema2pojo</groupId> <artifactId>jsonschema2pojo-maven-plugin</artifactId> <version>1.0.2</version> <configuration> <sourceDirectory>${basedir}/src/main/resources/schemas</sourceDirectory> <targetPackage>com.myproject.jsonschemas</targetPackage> <sourceType>json</sourceType> </configuration> <executions> <execution> <goals> <goal>generate</goal> </goals> </execution> </executions> </plugin>
For JSON sources, you can specify <sourceType>json</sourceType>. However, if you possess actual JSON schemas, this line is unnecessary.
In recent years, the JSON Schema specification has advanced significantly, offering a robust mechanism for defining structural rules. Furthermore, the jsonschema2pojo project provides a purpose-built tool that converts JSON schema documents into Java DTO classes. While still under development, it covers essential portions of the JSON schema. User feedback is crucial for its ongoing evolution, which you can provide through the command line or Maven plugin.
The above is the detailed content of How to Generate Java Classes from JSON Using the jsonschema2pojo Maven Plugin?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
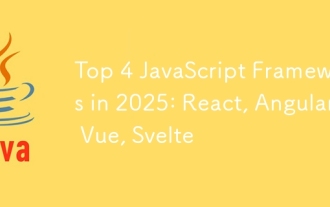
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
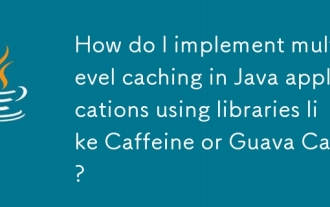
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
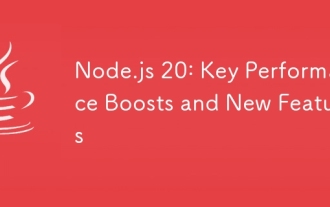
Node.js 20: Key Performance Boosts and New Features
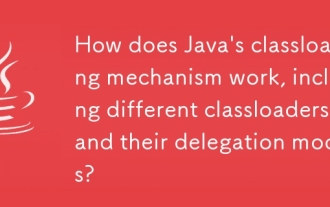
How does Java's classloading mechanism work, including different classloaders and their delegation models?
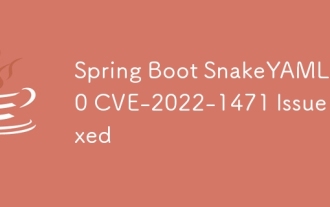
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
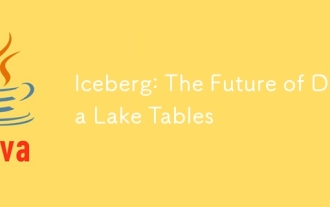
Iceberg: The Future of Data Lake Tables
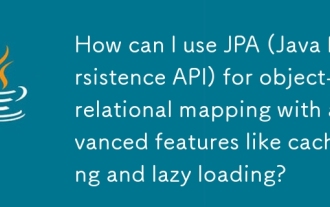
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
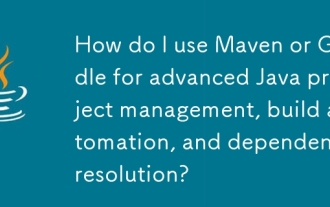
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
