How Can I Implement a HashMap to Store Multiple Values for the Same Key?
Implementing HashMap with Multiple Values for the Same Key
A HashMap is a data structure that maps keys to values. By default, each key can only have one associated value. However, it is possible to implement a HashMap that allows one key to have multiple values.
Options for Implementing Multiple Values:
1. Map with List as the Value:
Map
Use a map where the value is a list. This allows you to store multiple values under a single key. The disadvantage is that the list could contain more or less than two values.
2. Custom Wrapper Class:
Map
Create a wrapper class that contains the multiple values. This keeps the values bound to a single entity and provides encapsulation. However, it requires writing additional code to create and manage the wrapper class.
3. Tuple Class:
Map
Use a tuple class (if available in your programming language) to store the multiple values. This provides a simple and type-safe way to represent them.
4. Multiple Maps:
Map
Map
Use multiple maps to store the different values. While convenient, it can lead to disconnected values and maintenance issues if the maps get out of sync.
Examples:
Using a Map with List as the Value:
Map<String, List<Person>> peopleByForename = new HashMap<>(); List<Person> bobs = new ArrayList<>(); bobs.add(new Person("Bob Smith")); bobs.add(new Person("Bob Jones")); peopleByForename.put("Bob", bobs);
Using a Custom Wrapper Class:
class Wrapper { public Person person1; public Person person2; public Wrapper(Person person1, Person person2) { this.person1 = person1; this.person2 = person2; } } Map<String, Wrapper> peopleByForename = new HashMap<>(); peopleByForename.put("Bob", new Wrapper(new Person("Bob Smith"), new Person("Bob Jones")));
Using a Tuple Class:
// Assumes a Tuple2 class is available Map<String, Tuple2<Person, Person>> peopleByForename = new HashMap<>(); peopleByForename.put("Bob", Tuple2.create(new Person("Bob Smith"), new Person("Bob Jones")));
Using Multiple Maps:
Map<String, Person> firstPersonByForename = new HashMap<>(); Map<String, Person> secondPersonByForename = new HashMap<>(); firstPersonByForename.put("Bob", new Person("Bob Smith")); secondPersonByForename.put("Bob", new Person("Bob Jones"));
Consider the advantages and disadvantages of each approach before choosing the best option for your specific use case.
The above is the detailed content of How Can I Implement a HashMap to Store Multiple Values for the Same Key?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










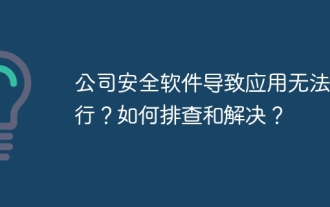
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
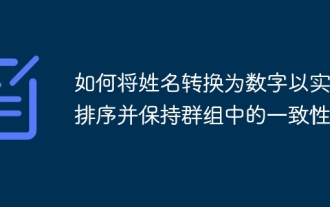
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
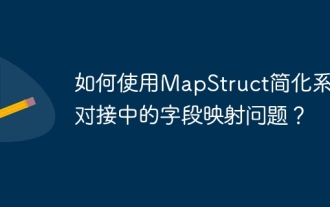
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
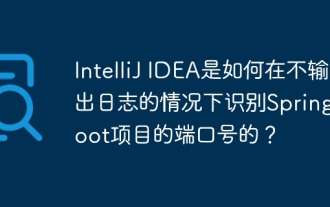
Start Spring using IntelliJIDEAUltimate version...
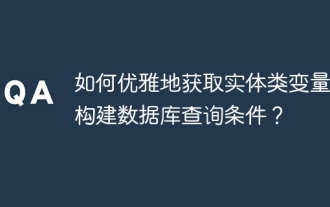
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
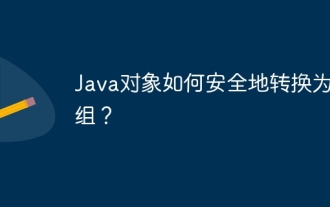
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
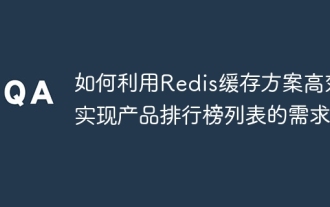
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
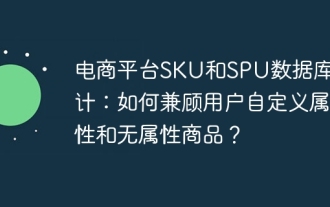
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
