


How Can I Efficiently Convert a Generic C Lambda to an std::function Using Templates?
Dec 09, 2024 am 11:03 AMLambda to std::function Conversion using Templates
In C , converting a lambda function to an std::function using templates can be a challenging task. To achieve this, template type deduction is essential, but it may face limitations in certain scenarios.
Initially, attempts to convert a lambda to std::function may fail due to missing template parameters or a mismatch in candidate matching. To resolve this, explicitly specifying the template parameters, as seen in std::function<void()> f([<unclosed>]), would suffice. However, this approach is not desirable for generic code that targets lambdas of any parameter type and count.
To address this issue, a more nuanced solution is required. While template type deduction cannot directly infer the std::function template parameters from a lambda, it can still be guided by providing an additional type constraint.
Consider the following approach: wrap the lambda function in a structure identity<std::function<void(T...)>>, where T can be deduced from the lambda parameters. This structure serves as an identity type, allowing the lambda to be passed as an argument without any type conversion.
template <typename T> struct identity { typedef T type; }; template <typename... T> void func(typename identity<std::function<void(T...)>>::type f, T... values) { f(values...); }
Now, when calling func, the template parameters of std::function can be deduced from the identity structure. This eliminates the need for explicit template parameter specification or additional argument passing.
int main() { func([](int x, int y, int z) { std::cout << (x*y*z) << std::endl; }, 3, 6, 8); return 0; }
This approach satisfies the requirements of converting a generic lambda to std::function without explicitly specifying the template parameters and allows the currying of variadic functions by preserving the original lambda signature.
The above is the detailed content of How Can I Efficiently Convert a Generic C Lambda to an std::function Using Templates?. For more information, please follow other related articles on the PHP Chinese website!

Hot tools Tags

Hot Article

Hot tools Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
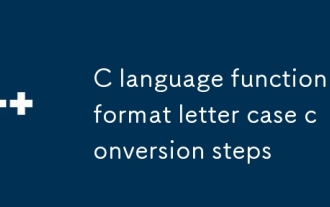
C language function format letter case conversion steps
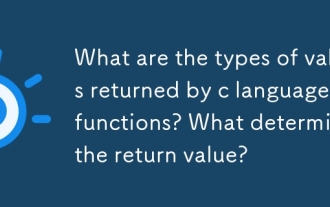
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
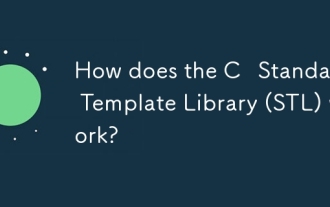
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?

What is the minimum common multiple of the maximum common divisor of a c language function?
