Logical Operators: When Should You Choose `||` Over `|`?
The Benefits of Logical Operators: Why Use || over |?
In programming, we often use logical operators to combine boolean expressions. The two most common logical operators are the logical OR operator (||) and the bitwise OR operator (|). While both operators perform similar functions, there is a key difference that makes || the preferred choice in most scenarios.
The bitwise OR operator (|) performs a bitwise operation, evaluating each bit of the two input booleans and returning the result bit by bit. In contrast, the logical OR operator (||) evaluates the input booleans sequentially, stopping as soon as one of them evaluates to true. This is known as short-circuiting.
Short-circuiting has several advantages over bitwise operation. First, it prevents unnecessary evaluation of the right-hand operand when the left-hand operand is already true. This can improve performance, especially when the right-hand operand is an expensive operation.
For example, consider the following code snippet:
Boolean b = true; if (b || foo.timeConsumingCall()) { // We entered without calling timeConsumingCall() }
Here, the use of || ensures that the function foo.timeConsumingCall() is not invoked, as the left-hand operand (b) is already true. This can be crucial when dealing with computationally expensive operations.
Another benefit of short-circuiting is null reference checks. If one of the input booleans is a null value, using && to check for falsiness will result in a NullPointerException, whereas || will evaluate to false without causing an error. For example:
String string = null; if (string != null && string.isEmpty()) { // We check for string being null before calling isEmpty() }
In this case, using && would throw a NullPointerException, while || would correctly evaluate to false.
In summary, while both || and | can be used for logical operations, the use of || is generally preferred due to its short-circuiting behavior. Short-circuiting improves performance and allows for safer null reference checks, making it the more suitable choice for most programming scenarios.
The above is the detailed content of Logical Operators: When Should You Choose `||` Over `|`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










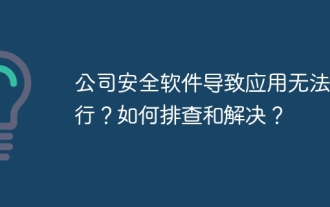
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
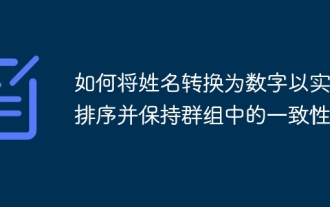
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
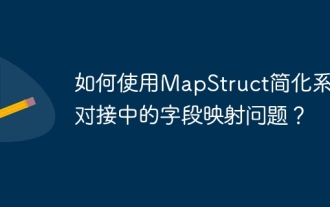
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
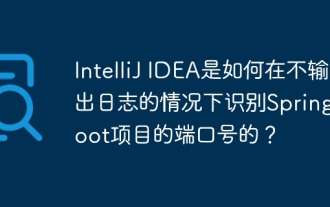
Start Spring using IntelliJIDEAUltimate version...
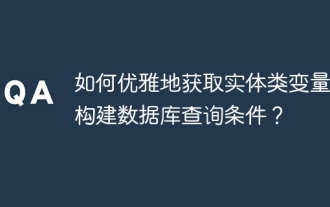
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
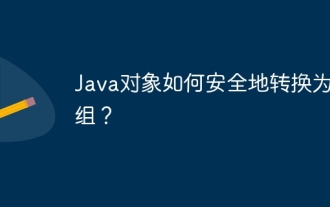
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
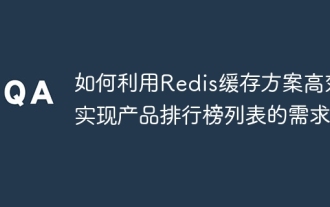
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
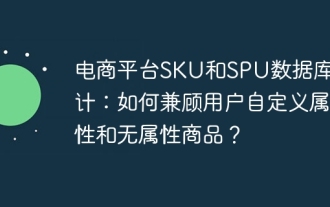
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
