


How Can I Scroll a Web Page Using Selenium Webdriver in Python?
Scrolling a Web Page using Selenium Webdriver in Python
In the realm of web automation, Selenium Webdriver stands as a trusted tool for navigating and interacting with web pages. One common challenge faced when automating tasks is scrolling down a web page to access additional content. This article delves into the various approaches to scrolling down using Selenium Webdriver in Python.
Approach 1: Specifying a Specific Height
To scroll to a specific height on the page, use the following syntax:
driver.execute_script("window.scrollTo(0, Y)")
where Y represents the desired height in pixels. For instance, to scroll down to a height of 1080 pixels (a full HD monitor's height), you would use:
driver.execute_script("window.scrollTo(0, 1080)")
Approach 2: Scrolling to the Bottom of the Page
To scroll to the very bottom of the page, execute the following code:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
This command ensures that you reach the end of the page, regardless of its actual height.
Approach 3: Infinite Scrolling
For web pages that employ infinite scrolling (e.g., social media feeds), you need to implement a custom scrolling mechanism:
SCROLL_PAUSE_TIME = 0.5 # Get the initial scroll height last_height = driver.execute_script("return document.body.scrollHeight") while True: # Scroll down to the bottom driver.execute_script("window.scrollTo(0, document.body.scrollHeight);") # Allow time for the page to load time.sleep(SCROLL_PAUSE_TIME) # Calculate the new scroll height and compare it with the previous one new_height = driver.execute_script("return document.body.scrollHeight") if new_height == last_height: break last_height = new_height
Additional Method: Selecting an Element and Scrolling
If you prefer, you can select an element on the page and scroll to it directly:
label = driver.find_element_by_css_selector("body") label.send_keys(Keys.PAGE_DOWN)
By selecting an element and sending the Keys.PAGE_DOWN command, the page will scroll down by one page.
The above is the detailed content of How Can I Scroll a Web Page Using Selenium Webdriver in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










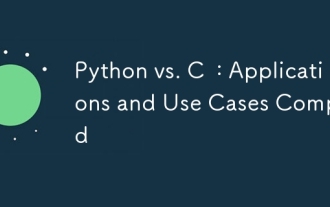
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
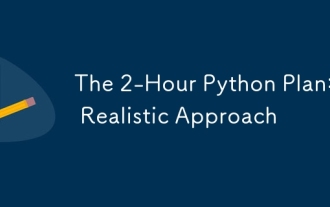
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
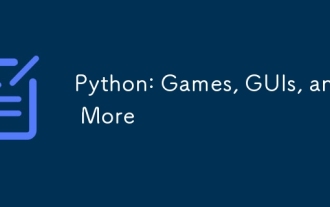
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
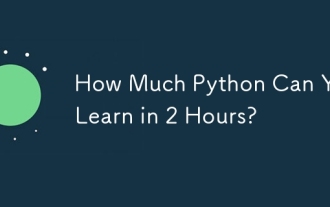
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
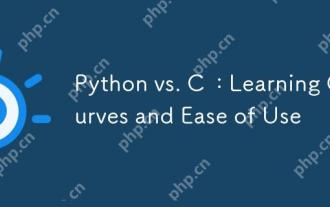
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
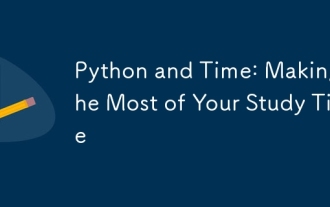
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
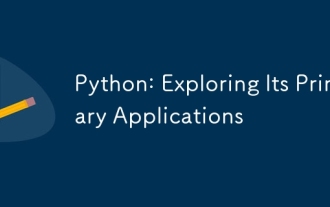
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
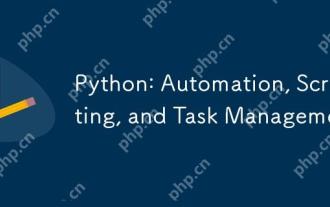
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
