


Is Using `list.List` the Best Way to Create a Go Map with String Keys and List Values?
Create a Map of String to List
Problem:
You want to create a Map with keys of type string and values of type List. Is the following code snippet the correct approach:
package main import ( "fmt" "container/list" ) func main() { x := make(map[string]*list.List) x["key"] = list.New() x["key"].PushBack("value") fmt.Println(x["key"].Front().Value) }
Answer:
The code snippet you provided does create a Map of string to List, but it may not be the most efficient approach. When working with Lists in Go, slices are generally a more suitable choice due to their performance advantages.
Using Slices:
The following code snippet demonstrates how to use slices instead of Lists:
package main import "fmt" func main() { x := make(map[string][]string) x["key"] = append(x["key"], "value") x["key"] = append(x["key"], "value1") fmt.Println(x["key"][0]) fmt.Println(x["key"][1]) }
Benefits of Using Slices:
Slices offer several advantages over Lists, including:
- Performance: Slices are more efficient when accessing and modifying elements compared to Lists.
- Ease of Use: Slices have a simpler syntax, making them easier to work with.
- Built-In Functions: Slices provide a wide range of built-in functions for operations such as sorting, searching, and slicing.
The above is the detailed content of Is Using `list.List` the Best Way to Create a Go Map with String Keys and List Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










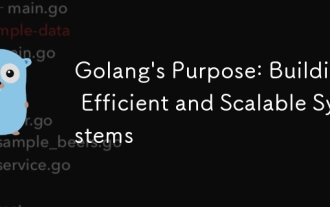
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
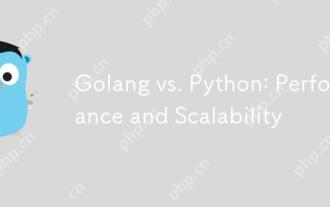
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
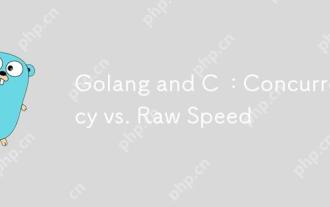
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
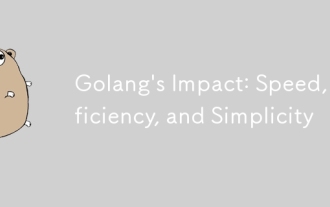
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
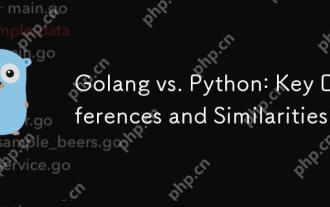
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
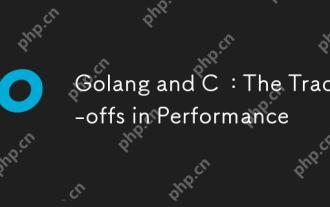
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
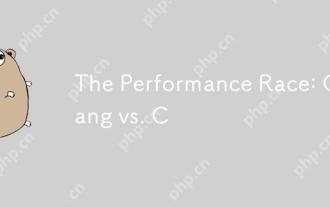
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
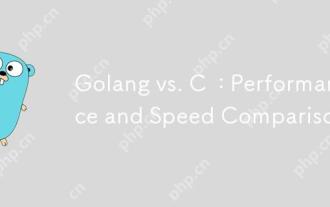
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
