


How Do Nullable Types Work in PHP, and What Are the Syntax Rules?
Nullable Types in PHP 7: Understanding ? Before Type Declaration
Nullable types are a powerful feature introduced in PHP 7.1 that allow you to declare variables, parameters, and return values as nullable. This signifies that they can accept or return either the specified type or NULL.
Nullable Parameters
Using a question mark (?) before the type declaration in function parameters indicates that the parameter can be either the specified type or NULL. For example:
public function (?string $parameter1, string $parameter2) {}
In this example, both parameters are nullable. You can pass either a string value or NULL as an argument to the function.
Nullable Return Types
Similarly, a function's return type can be declared as nullable using the question mark syntax. This indicates that the function can return either the specified type or NULL. For example:
function error_func(): int { return null ; // Uncaught TypeError: Return value must be of the type integer } function valid_func(): ?int { return null ; // OK }
Nullable Property Types (PHP 7.4 )
Starting with PHP 7.4, you can also declare property types as nullable. This allows for properties to have either the specified type or NULL. For example:
class Foo { private ?object $bar = null; // OK : can be null (nullable type) }
Nullable Union Types (PHP 8 )
PHP 8.0 introduced a shorthand notation for nullable union types. Writing "?T" is equivalent to "T|null". This allows for more concise type declarations.
class Foo { private object|null $baz = null; // as of PHP 8.0 }
Syntax Error in PHP Versions Lower than 7.1
If you try to use the question mark syntax with PHP versions lower than 7.1, you will encounter a syntax error. In such cases, you should remove the question mark.
Conclusion
Nullable types provide a convenient way to handle nullable values in PHP. They allow you to declare that a variable, parameter, or return value can be either a specific type or NULL, making your code more robust and easier to maintain.
The above is the detailed content of How Do Nullable Types Work in PHP, and What Are the Syntax Rules?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


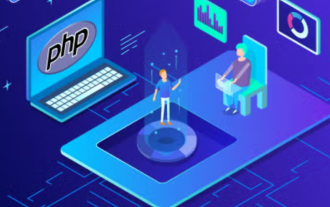
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
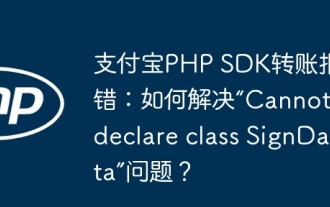
Alipay PHP...
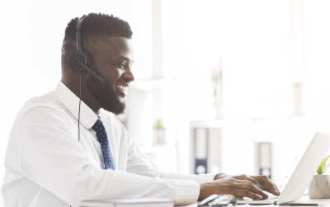
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
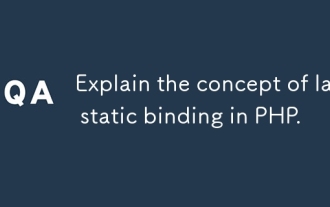
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
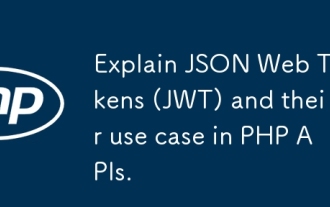
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
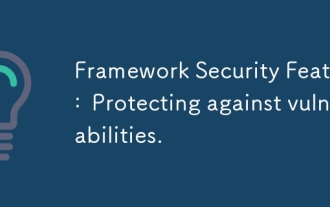
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
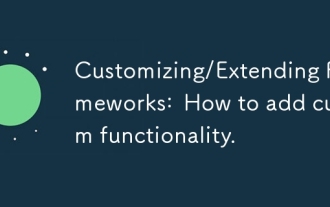
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
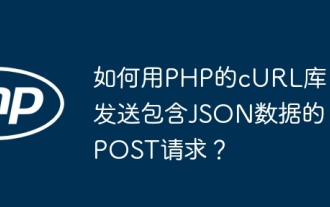
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
