


How Can I Efficiently Calculate the Cumulative Sum of a List in Python?
Dec 09, 2024 pm 03:56 PMCumulative Sum in Python
Question:
How can we find the cumulative sum of numbers in a list, like [4, 4 6, 4 6 12]?
Introduction:
Calculating the cumulative sum involves repeatedly adding the next element to the running sum. While this can be achieved with a straightforward loop, there are more efficient approaches using NumPy or custom Python generators.
NumPy Method:
NumPy's cumsum function provides an efficient solution:
import numpy as np time_interval = [4, 6, 12] np.cumsum(time_interval) # Output: [4, 10, 22]
This approach is highly optimized and recommended for numerical operations on large arrays.
Python Generator:
For concise and memory-efficient solutions in pure Python, consider using a generator:
def accumu(iterable): yield next(iterable) for item in iterable: yield item + sum(accumu(iterable))
Using this generator:
list(accumu(time_interval)) # Output: [4, 10, 22]
This generator lazily accumulates elements, making it suitable for iterating over large sequences or when memory is a concern.
The above is the detailed content of How Can I Efficiently Calculate the Cumulative Sum of a List in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
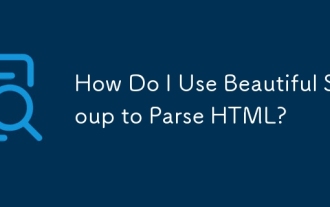
How Do I Use Beautiful Soup to Parse HTML?
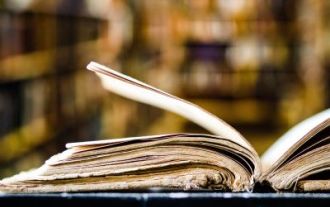
How to Use Python to Find the Zipf Distribution of a Text File
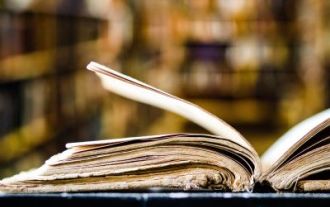
How to Work With PDF Documents Using Python
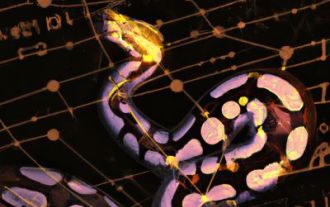
How to Cache Using Redis in Django Applications
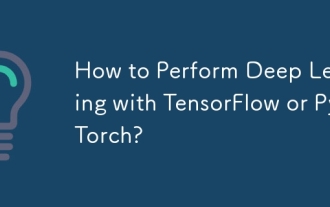
How to Perform Deep Learning with TensorFlow or PyTorch?
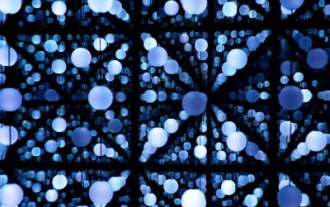
How to Implement Your Own Data Structure in Python
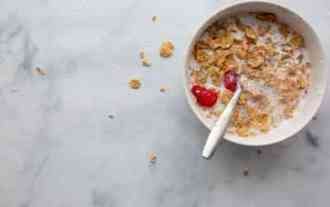
Serialization and Deserialization of Python Objects: Part 1
