Understanding the map() Method for JavaScript Arrays: A Simple Guide
The map() method creates a new array by applying a provided function (callbackFn) to each element of the original array. It’s perfect for transforming data without modifying the original array.
Syntax
array.map(callbackFn, thisArg)
-
callbackFn: A function that runs on each array element, with the following arguments:
- element: Current element.
- index: Current index.
- array: The array being traversed.
- thisArg (optional): A value to use as this in the callback function.
Key Features
- Returns a New Array: The original array remains unchanged.
- Skips Empty Slots: Callback is not called for unassigned elements in sparse arrays.
- Generic Usage: Works with array-like objects (e.g., NodeLists).
Examples
1. Basic Example: Transform Numbers
const numbers = [1, 4, 9]; const roots = numbers.map((num) => Math.sqrt(num)); console.log(roots); // [1, 2, 3]
2. Reformat Objects
const kvArray = [ { key: 1, value: 10 }, { key: 2, value: 20 }, ]; const reformatted = kvArray.map(({ key, value }) => ({ [key]: value })); console.log(reformatted); // [{ 1: 10 }, { 2: 20 }]
3. Using parseInt with map
// Common mistake: console.log(["1", "2", "3"].map(parseInt)); // [1, NaN, NaN] // Correct approach: console.log(["1", "2", "3"].map((str) => parseInt(str, 10))); // [1, 2, 3] // Alternative: console.log(["1", "2", "3"].map(Number)); // [1, 2, 3]
4. Avoid Undefined Results
Returning nothing from the callback leads to undefined in the new array:
const numbers = [1, 2, 3, 4]; const result = numbers.map((num, index) => (index < 3 ? num : undefined)); console.log(result); // [1, 2, 3, undefined]
Use filter() or flatMap() to remove undesired elements.
5. Side Effects (Anti-Pattern)
Avoid using map() for operations with side effects, like updating variables:
const cart = [5, 15, 25]; let total = 0; // Avoid this: const withTax = cart.map((cost) => { total += cost; return cost * 1.2; }); // Instead, use separate methods: const total = cart.reduce((sum, cost) => sum + cost, 0); const withTax = cart.map((cost) => cost * 1.2);
6. Accessing Other Array Elements
The third argument (array) allows accessing neighbors during transformations:
const numbers = [3, -1, 1, 4]; const averaged = numbers.map((num, idx, arr) => { const prev = arr[idx - 1] || 0; const next = arr[idx + 1] || 0; return (prev + num + next) / 3; }); console.log(averaged);
Common Use Cases
- Transform Data: Apply a function to each element.
- Reformat Objects: Change the structure of data.
- Map NodeLists: Transform DOM elements like NodeList into arrays:
const elems = document.querySelectorAll("option:checked"); const values = Array.from(elems).map(({ value }) => value);
When to Avoid map()
- No Return Value Needed: Use forEach() or for...of instead.
- Mutating Data: Create new objects instead of altering the original ones:
const products = [{ name: "phone" }]; const updated = products.map((p) => ({ ...p, price: 100 }));
Final Tips
- Pure Functions Only: Ensure the callback has no side effects.
- Understand Arguments: Know that map() passes element, index, and array to the callback.
- Avoid Sparse Arrays: Empty slots will remain empty.
Use map() to simplify your code when transforming arrays efficiently!
The above is the detailed content of Understanding the map() Method for JavaScript Arrays: A Simple Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


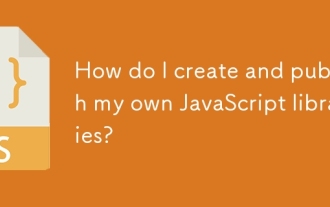
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
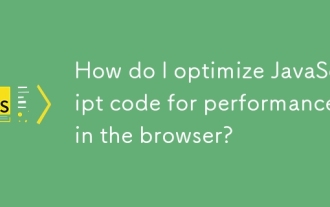
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
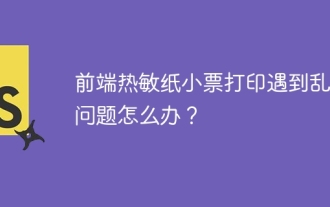
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
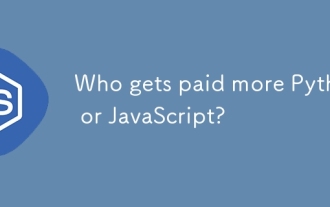
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
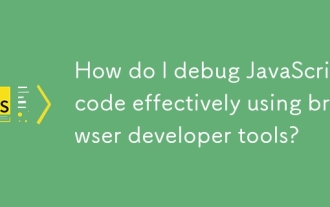
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
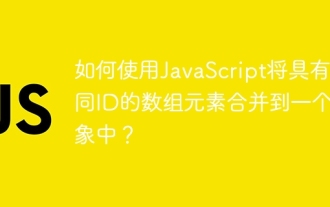
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
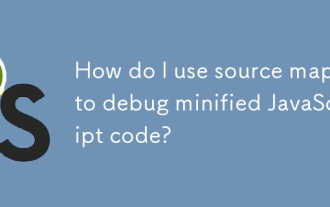
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
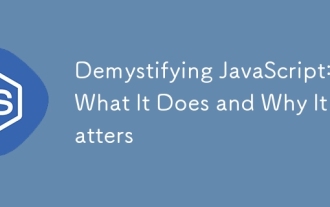
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
