


How to Efficiently Find a Key in a PHP Multidimensional Array by its Value?
PHP Multidimensional Array Searching (Find Key by Value)
In this scenario, you have a multidimensional array representing a collection of products. Each product is defined by properties such as name, slug, price, etc. Your goal is to find a way to search the array for a specific product using the slug property and return its corresponding key in the array.
One way to achieve this is by leveraging the array_search function and the array_column function. Here's a custom function that encapsulates this approach:
function array_search_multidim(array $array, string $column, string $key) { return array_search($key, array_column($array, $column)); }
To use this function, you would call it like so:
$product_key = array_search_multidim($products, 'slug', 'breville-one-touch-tea-maker-BTM800XL');
This will search the $products array for a product with the slug property equal to 'breville-one-touch-tea-maker-BTM800XL' and return its corresponding key. In this example, it will return the value 1.
Another possible solution is based on the array_search function. For this approach, you need to use PHP 5.5.0 or higher:
$key = array_search('breville-one-touch-tea-maker-BTM800XL', array_column($products, 'slug'));
This code achieves the same result as the previous example.
There is also a third method using array_walk_recursive that recursively goes through all the elements of the array and compares the value of the slug with the given value:
function array_walk_recursive_search($input, $needle, &$result = null) { if (is_array($input)) { foreach ($input as $key => $value) { array_walk_recursive_search($value, $needle, $result); } } else { if ($input === $needle) { $result = $key; } } }
This function can be invoked as follows:
array_walk_recursive($products, 'breville-one-touch-tea-maker-BTM800XL', $product_key);
It's important to note that while the array_walk_recursive method is more general and can handle any type of array structure, it is generally slower than the other methods.
Overall, the best method to use will depend on the specific needs and constraints of your application. However, the two most efficient methods are the ones that utilize the array_search and array_column functions.
The above is the detailed content of How to Efficiently Find a Key in a PHP Multidimensional Array by its Value?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










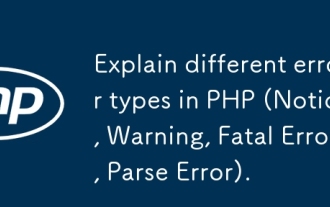
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
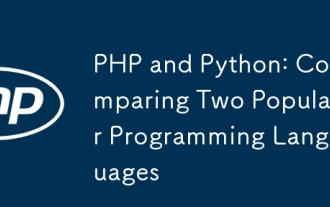
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
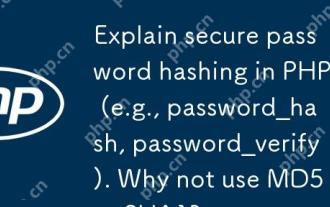
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
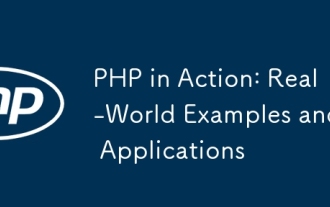
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
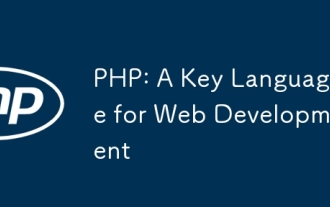
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
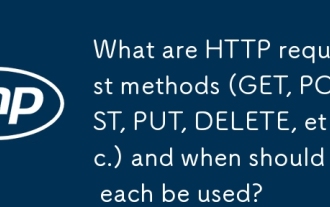
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
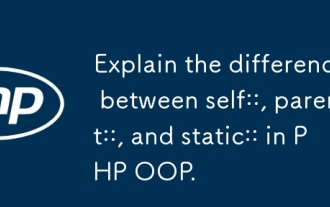
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
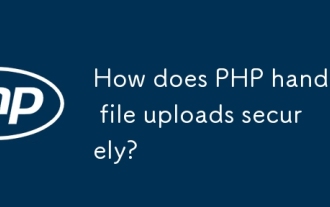
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
