How to Efficiently Display Firebase Data in an Android ListView?
Displaying Firebase Data in ListView
One common challenge developers face pertains to displaying data from Firebase in a ListView. Recently, a user posted a question about this topic, highlighting issues encountered. Let's dive into their concerns and provide solutions.
The Issue
Their code initially displayed User ID but failed to show the score. Upon making some changes, they were left with null values. Suspecting a deletion or misspelling issue, they sought assistance.
Addressing the Problem
Upon reviewing the provided code, the issue stemmed from not following Java Naming Conventions in the model class. The class should be named "Score" and formatted as follows:
public class Score { private String userId, score; public Score() {} public Score(String userId, String score) { this.userId = userId; this.score = score; } public String getUserId() { return userId; } public String getScore() { return score; } @Override public String toString() { return "Score{" + "userId='" + userId + '\'' + ", score='" + score + '\'' + '}'; } }
Additionally, the onDataChange() method's asynchronous nature caused the list to remain empty. To remedy this, the list declaration should be moved inside onDataChange().
Asynchronous Behavior and Data Retrieval
It's important to understand the asynchronous behavior of the onDataChange() method. In this case, it's called even before attempting to add objects to the list. Consequently, any attempt to use the list outside of that method will yield empty results.
To address this behavior, the list declaration can be moved inside onDataChange(), as seen in the following code:
dbref.addValueEventListener(new com.google.firebase.database.ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { ArrayList<String> list = new ArrayList<>(); for(DataSnapshot ds :dataSnapshot.getChildren()) { Score Result = ds.getValue(Score.class); String userId = String.valueOf(Result.getUserId()); String score = String.valueOf(Result.getScore()); list.add(userId); list.add(score); } adapter = new ArrayAdapter<>(getActivity(), android.R.layout.simple_list_item_1, list); LvRanking.setAdapter(adapter); }
Displaying Data Using the String Class
To display the data using the String class, you can use the following code:
ListView listView = findViewById(R.id.list_view); List<String> list = new ArrayList<>(); ArrayAdapter<String> arrayAdapter = new ArrayAdapter<>(getActivity(), android.R.layout.simple_list_item_1, list); listView.setAdapter(arrayAdapter); DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference(); DatabaseReference scoreRef = rootRef.child("score"); ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { for(DataSnapshot ds : dataSnapshot.getChildren()) { String userId = ds.child("userId").getValue(String.class); String score = ds.child("score").getValue(String.class); list.add(userId + " / " + score); Log.d("TAG", userId + " / " + score); } arrayAdapter.notifyDataSetChanged(); } @Override public void onCancelled(DatabaseError databaseError) { Log.d(TAG, task.getException().getMessage()); } }; scoreRef.addListenerForSingleValueEvent(eventListener);
Displaying Data Using the Score Class
Alternatively, to display data using the Score class, use the following code:
ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { for(DataSnapshot ds : dataSnapshot.getChildren()) { Score score = ds.getValue(Score.class); String userId = score.getUserId(); String score = score.getScore(); Log.d("TAG", userId + " / " + score); list.add(score); } arrayAdapter.notifyDataSetChanged(); } @Override public void onCancelled(DatabaseError databaseError) { Log.d(TAG, task.getException().getMessage()); } }; scoreRef.addListenerForSingleValueEvent(eventListener);
By following these solutions, you should be able to successfully display Firebase data in a ListView.
The above is the detailed content of How to Efficiently Display Firebase Data in an Android ListView?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
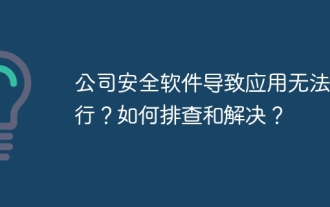
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
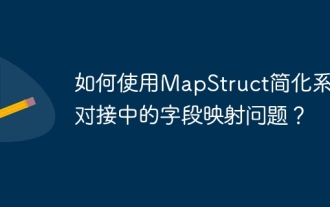
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
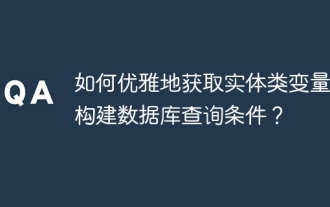
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
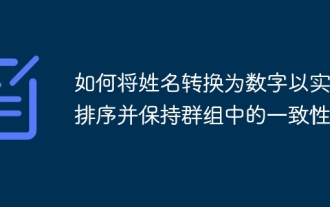
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
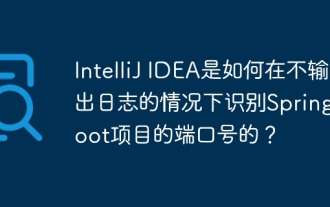
Start Spring using IntelliJIDEAUltimate version...
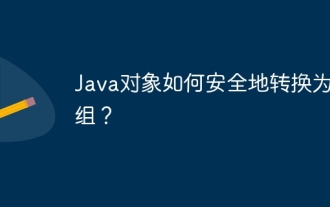
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
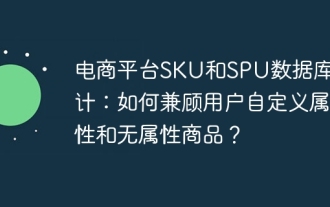
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
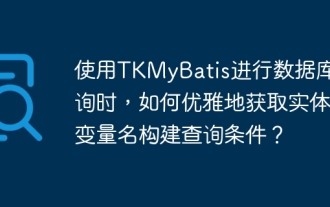
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
