


How to Effectively Monitor and Control the Number of Active Goroutines in Go?
How to Display the Number of Active Goroutines
In a scenario where you have a queue with concurrent operations, it becomes essential to monitor the number of active goroutines. This ensures that the appropriate number of goroutines are handling the queue, optimizing performance and resource utilization.
The provided code snippet demonstrates a loop that spawns goroutines based on the number of elements in a queue. However, this approach has several drawbacks:
- It keeps spawning goroutines indefinitely.
- It can lead to excessive CPU usage due to redundant processing.
A better solution is to utilize a synchronization mechanism. Here, a WaitGroup is employed:
func deen(wg *sync.WaitGroup, queue chan int) { for element := range queue { wg.Done() fmt.Println("element is ", element) if element%2 == 0 { fmt.Println("new element is ", element) wg.Add(2) queue <- (element*100 + 11) queue <- (element*100 + 33) } } } func main() { var wg sync.WaitGroup queue := make(chan int, 10) queue <- 1 queue <- 2 queue <- 3 queue <- 0 for i := 0; i < 4; i++ { wg.Add(1) go deen(&wg, queue) } wg.Wait() close(queue) fmt.Println("list len", len(queue)) //this must be 0 }
This revised code ensures that the number of goroutines is always limited to four, and it completes processing once all elements in the queue have been handled. This approach effectively addresses the problem of excessive goroutine creation and CPU consumption.
The above is the detailed content of How to Effectively Monitor and Control the Number of Active Goroutines in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


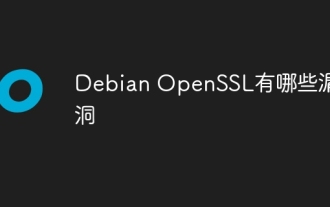
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
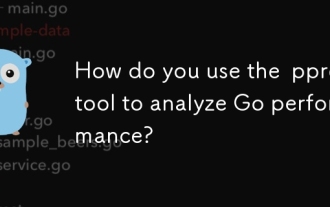
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
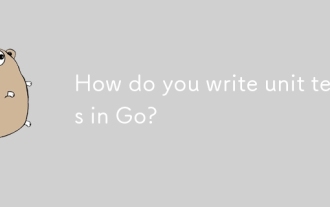
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
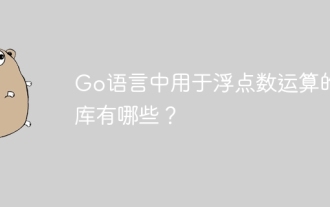
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
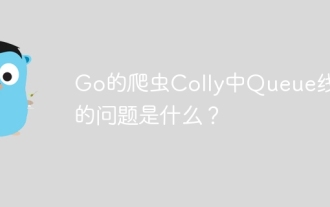
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
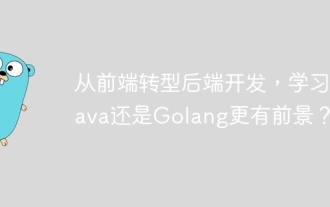
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
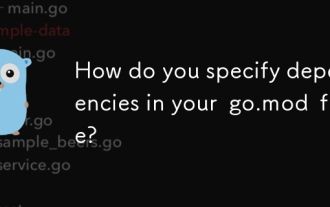
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
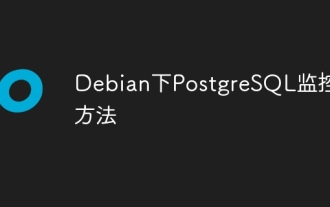
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
