


How Can I Efficiently Use Go Reflection to Call the `Scan` Variadic Function with a Dynamic Number of Pointers?
Calling Scan Variadic Function with Reflection in Go
The Scan() method of Row interface in the database/sql package allows efficient retrieval of values from a database row by passing a variadic list of pointers to values of correct type. While useful, working with this method using reflection to obtain values from a variable number of pointers can be challenging.
Solution
The provided solution overcomes this challenge by dynamically creating two slices: one for storing values and one for holding parallel pointers. Using pointers to collect data in the values slice enables the population of other data structures.
Code
package main import ( "fmt" _ "github.com/lib/pq" "database/sql" ) func main() { db, _ := sql.Open( "postgres", "user=postgres dbname=go_testing password=pass sslmode=disable") rows, _ := db.Query("SELECT * FROM _user;") columns, _ := rows.Columns() count := len(columns) values := make([]interface{}, count) valuePtrs := make([]interface{}, count) for rows.Next() { for i := range columns { valuePtrs[i] = &values[i] } rows.Scan(valuePtrs...) for i, col := range columns { val := values[i] b, ok := val.([]byte) var v interface{} if (ok) { v = string(b) } else { v = val } fmt.Println(col, v) } } }
The code first opens a connection to the database and executes a query. It then determines the number of columns in the result set and creates two slices, values and valuePtrs, with the same length as the columns.
Inside the loop over the rows, the valuePtrs slice is populated with the addresses of each element in the values slice. The rows.Scan() method is then called with the valuePtrs slice, effectively populating the values slice with the data from the current row.
Finally, the code iterates over the column names and values, converting byte slices to strings if necessary, and printing the results.
The above is the detailed content of How Can I Efficiently Use Go Reflection to Call the `Scan` Variadic Function with a Dynamic Number of Pointers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










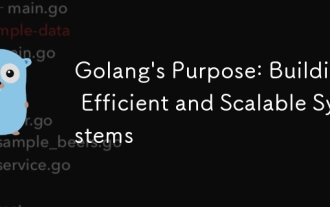
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
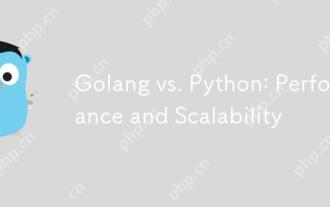
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
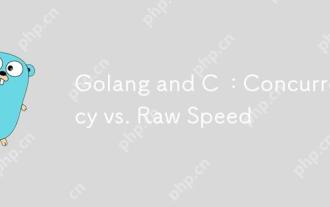
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
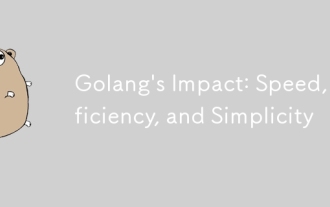
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
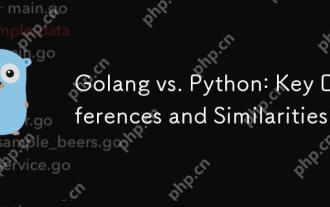
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
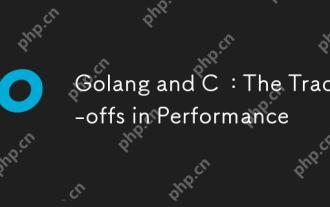
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
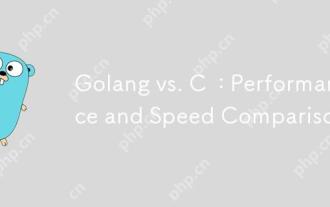
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
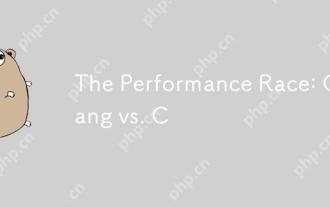
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
