


How can I convert a backslash-delimited string into an associative array in PHP?
Convert Backslash-Delimited String into an Associative Array
In PHP, a common task is to process strings formatted with key-value pairs separated by a separator. A common example is a backslash-delimited string, where key and value pairs are separated by a backslash ().
Using preg_match_all and array_combine
One effective method involves using the preg_match_all function to extract both keys and values into separate arrays, which are then combined using array_combine.
preg_match_all("/([^\\]+)\\([^\\]+)/", $string, $p); $array = array_combine($p[1], $p[2]);
This regex pattern matches any non-backslash characters into $p[1] (keys) and any non-backslash characters into $p[2] (values).
Customizing Key/Value Separators
This approach can be generalized to handle different key-value separators:
preg_match_all("/ ([^:]+) : ([^,]+) /x", $string, $p); $array = array_combine($p[1], $p[2]);
Simply replace ":" with your desired key-value separator and "," with your desired pair delimiter.
Allowing Varying Delimiters
To allow varying delimiters, use:
preg_match_all("/ ([^:=]+) [:=]+ ([^,+&]+) /x", $string, $p);
This permits key=value, key2:value2, or similar variations.
Additional Features
You can further enhance the extraction:
- Constrain Alphanumeric Keys: Ensure keys are alphanumeric.
- Strip Spaces/Quoting: Remove unnecessary spaces or quotes.
- INI-Style Extraction: Extract data in the common INI format (key=value).
Alternative: parse_str
For convenient handling of key=value&key2=value2 strings, consider using parse_str with strtr:
parse_str(strtr($string, ":,", "=&"), $pairs);
Considerations
- preg_match_all array_combine: Highly flexible, customizable, and well-suited for unknown delimiters.
- parse_str: Simpler and optimized for known delimiters like "&".
- explode foreach: Manual approach, often slower and more verbose.
Choose the most appropriate method based on your requirements and trade-offs.
The above is the detailed content of How can I convert a backslash-delimited string into an associative array in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










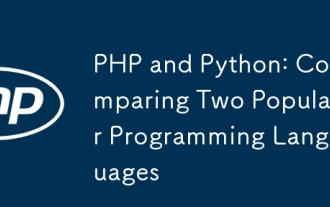
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
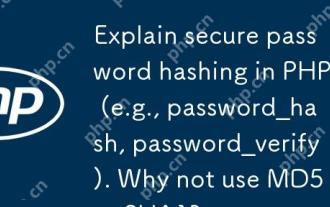
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
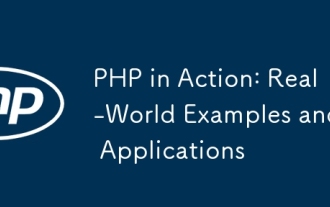
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
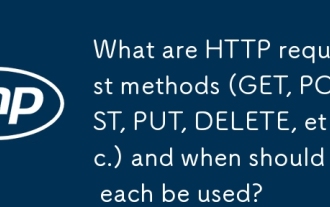
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
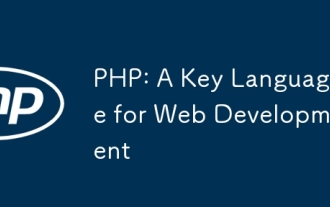
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
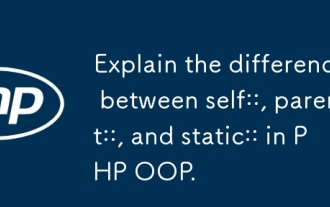
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
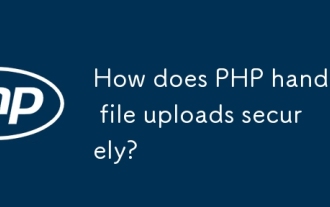
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
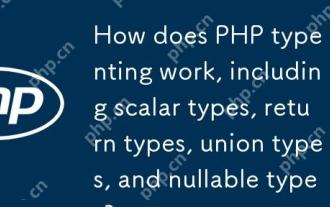
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
