How to Write Effective Unit Tests in Java Using JUnit?
How to Write a Unit Test in Java
Unit testing is an essential software development practice that involves testing individual units of code. In Java, you can use unit testing frameworks such as JUnit to create tests that verify the behavior of your classes and methods.
Creating Unit Tests in Eclipse
- Create a new Java project.
- Right-click on your project and create a new class.
- Write your code in the class.
- Right-click on your project and create a new JUnit Test Case.
- Initialize your test in the setUp() method.
- Create a test method and write your assertions.
- Run the test by right-clicking on your test class and selecting "Run as" -> "JUnit Test".
Creating Unit Tests in IntelliJ
- Create a directory called 'test' in your project's main folder.
- Mark the test directory as a "test sources root".
- Create a Java test class in the appropriate package within the test directory.
- Add the JUnitx dependency to your class.
- Write your test method with the signature: @Test public void test
(). - Write your assertions using methods like Assertions.assertTrue().
- Run the test by right-clicking on your test class and selecting "Run".
Example: Testing a Binary Sum Class
Here's an example of a Java class that performs a binary sum and its corresponding unit test in JUnit:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
This test verifies the correctness of the BinarySum class by comparing the expected result with the actual result. By running these unit tests, you can ensure that your code is working as intended before integrating it into your application.
The above is the detailed content of How to Write Effective Unit Tests in Java Using JUnit?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
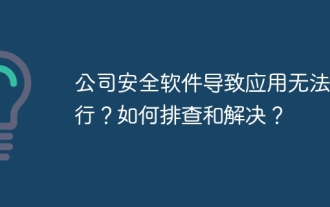
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
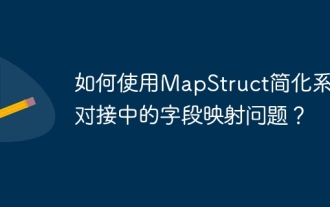
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
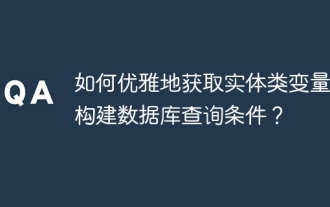
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
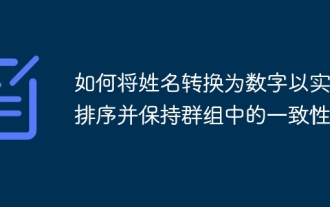
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
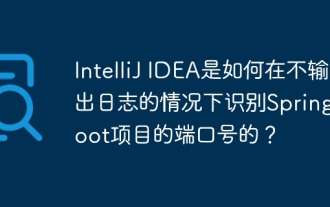
Start Spring using IntelliJIDEAUltimate version...
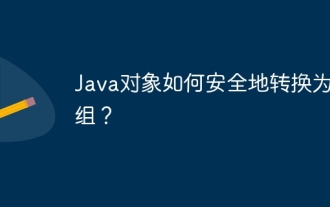
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
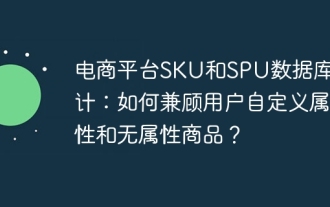
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
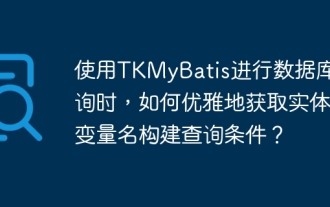
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
