


How to Read Space-Separated Values from a Text File into an ArrayList in Java?
Dec 10, 2024 am 07:15 AMReading Space-Separated Values from a Text File into an ArrayList
Reading space-separated values from a text file and storing them in an array list involves several steps in Java.
Firstly, use Files#readAllLines() to retrieve all lines in the text file and store them in a List<String>:
List<String> lines = Files.readAllLines(Paths.get("/path/to/file.txt"));
Next, split each line into individual values using String#split() with the whitespace character as the delimiter:
for (String line : lines) { String[] parts = line.split("\s+"); }
To convert these string values to integers, use Integer#valueOf():
List<Integer> numbers = new ArrayList<>(); for (String part : parts) { numbers.add(Integer.valueOf(part)); }
Once all values are converted to integers and added to the array list, you can access and use them as desired. This approach ensures accurate and efficient reading of space-separated values from a text file into an array list.
The above is the detailed content of How to Read Space-Separated Values from a Text File into an ArrayList in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
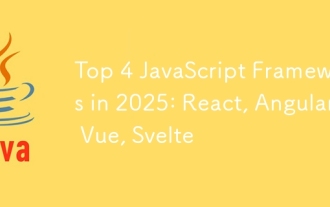
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
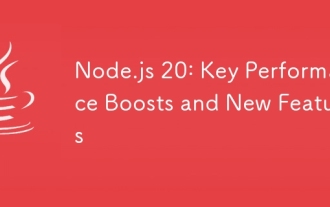
Node.js 20: Key Performance Boosts and New Features
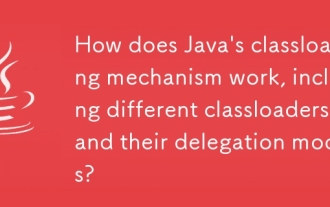
How does Java's classloading mechanism work, including different classloaders and their delegation models?
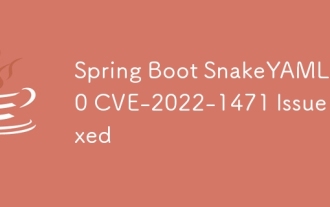
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
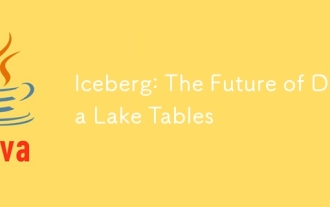
Iceberg: The Future of Data Lake Tables
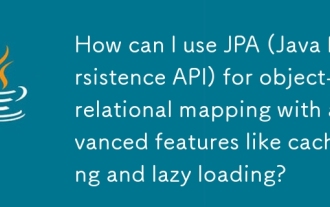
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
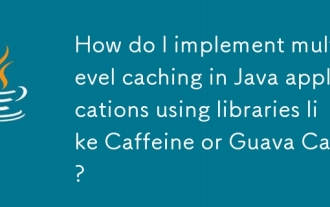
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
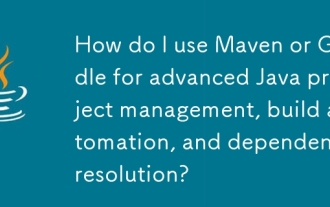
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
