How Do Arrow Functions Handle the `this` Keyword in JavaScript?
Dec 10, 2024 am 10:19 AMArrow Functions and 'this'
In ES6, arrow functions introduce a new approach to method definition. However, there's a notable difference between arrow functions and traditional functions when it comes to accessing the 'this' keyword.
The Issue:
Consider the following code:
var person = { name: "jason", shout: () => console.log("my name is ", this.name) } person.shout() // Should print out my name is jason
While the intended outcome is to print "my name is jason," the console only outputs "my name is." This is because arrow functions behave differently from traditional functions in terms of 'this' binding.
The Explanation:
Unlike traditional functions, arrow functions do not bind the 'this' keyword to the containing scope. Instead, they inherit the 'this' binding from the surrounding context. In the example above, the 'this' in the arrow function refers to the global object, not the 'person' object.
The Solution:
There are several ways to address this issue:
- Use a Bound Function:
// Bind 'this' to the 'person' object var shoutBound = function() { console.log("my name is ", this.name); }.bind(person); // Assign the bound function to the 'shout' property person.shout = shoutBound;
- Use a Traditional Function:
// Define a traditional function with 'this' bound to 'person' person.shout = function() { console.log("my name is ", this.name); };
- Use ES6 Method Declaration:
// ES6 method declaration implicitly binds 'this' to the object person = { name: "jason", shout() { console.log("my name is ", this.name); } };
By understanding the different behaviors of arrow functions regarding 'this' binding, you can write effective and flexible code in ES6.
The above is the detailed content of How Do Arrow Functions Handle the `this` Keyword in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
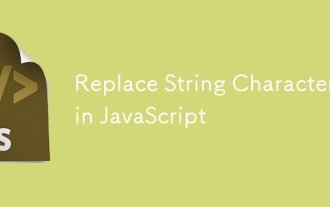
Replace String Characters in JavaScript
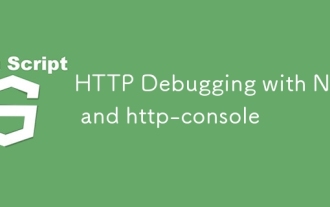
HTTP Debugging with Node and http-console
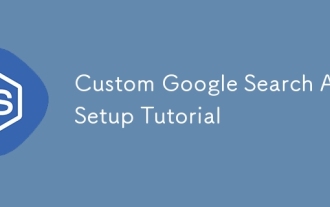
Custom Google Search API Setup Tutorial
