How Can Java's Map.Entry and SimpleEntry Simplify Value Pair Management?
A Comprehensive Collection for Value Pairs: Introducing Java's Map.Entry and SimpleEntry
In Java, when defining a collection where each element comprises a pair of values with distinct types, a Map is commonly employed. However, for scenarios where maintaining the order of elements is crucial and uniqueness is not a concern, there's a valuable alternative: Java's Map.Entry interface and its implementation, SimpleEntry.
Map.Entry and SimpleEntry: A Pair Perfection
Map.Entry provides a framework for representing an individual key-value association within a Map. It possesses two generic type parameters,
Creating and Utilizing Value Pairs
To establish a pair of values, instantiate a SimpleEntry object, specifying the types for the key and value. For instance:
Map.Entry<String, Integer> pair1 = new SimpleEntry<>("Key 1", 10);
Alternately, you can leverage Java's varargs (variable-length arguments) feature to create multiple pairs in a single line:
var pairList = List.of( new SimpleEntry<>("Key 1", 10), new SimpleEntry<>("Key 2", 20) );
Exploiting Generics and Subclassing
To further enhance readability and maintainability, you can embrace generics and subclassing. Create a TupleList class that extends ArrayList
class TupleList<T extends Map.Entry<K, V>> extends ArrayList<T> { public TupleList<T> of(K key, V value) { add(new SimpleEntry<>(key, value)); return this; } }
With this elegant extension, you can swiftly construct value pairs using an intuitive syntax:
TupleList<Map.Entry<String, Integer>> pair = new TupleList<>(); pair.of("Key 1", 10); pair.of("Key 2", 20);
Conclusion
Java's Map.Entry and SimpleEntry provide a versatile mechanism for storing and manipulating value pairs. Their flexibility and ease of use make them an excellent choice for scenarios where order and distinct types play a significant role. By leveraging generics and subclassing techniques, you can further simplify your code and achieve a level of code clarity that belies their underlying complexity.
The above is the detailed content of How Can Java's Map.Entry and SimpleEntry Simplify Value Pair Management?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










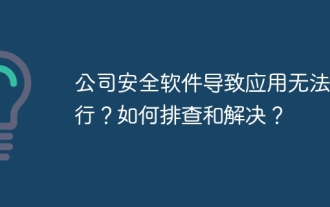
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
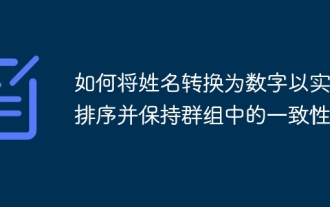
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
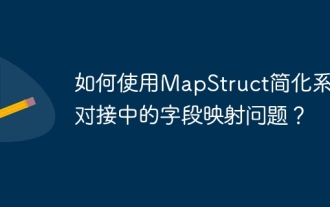
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
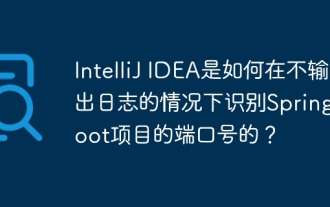
Start Spring using IntelliJIDEAUltimate version...
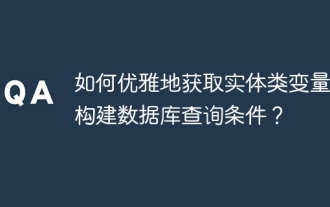
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
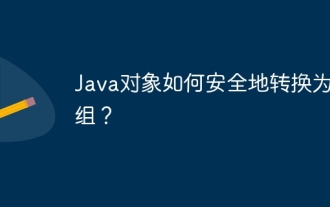
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
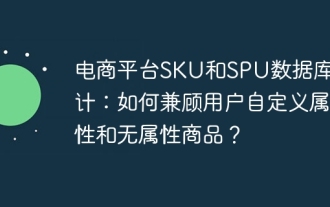
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
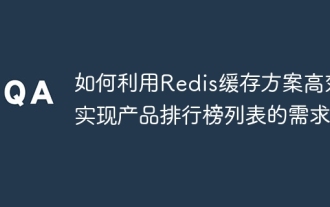
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
