


How to Efficiently Chain Processes Using Python's `subprocess.Popen` with or without Pipes?
How to Chain Multiple Processes with Pipes Using subprocess.Popen
In Python, the subprocess module provides a convenient interface for interacting with the operating system's processes. When dealing with complex command pipelines, it's possible to encounter challenges in connecting multiple processes.
Defining the Problem
Suppose we wish to execute the following shell command using subprocess.Popen:
echo "input data" | awk -f script.awk | sort > outfile.txt
However, we have an input string instead of using echo. Moreover, we need guidance on how to pipe the output of the awk process to sort.
Solution with Pipes
import subprocess # Delegate the pipeline to the shell awk_sort = subprocess.Popen("awk -f script.awk | sort > outfile.txt", stdin=subprocess.PIPE, shell=True) awk_sort.communicate(b"input data\n")
This approach leverages the shell's capabilities to create the pipeline while Python is responsible for providing input and capturing output.
Avoiding Pipes with Python
An alternative solution involves rewriting the script.awk script in Python, eliminating the need for both awk and the pipeline. This approach simplifies the code and eliminates potential compatibility issues.
Reasons to Avoid Awk
The answer also suggests reasons why using awk may not be the most optimal solution:
- Redundancy: awk adds an unnecessary processing step. Python can handle the required processing.
- Performance: Pipelining large datasets may benefit from concurrency, but for small datasets, it's negligible.
- Simplicity: Python-to-sort processing is more straightforward and avoids potential complexities.
- Programming language diversity: Eliminating awk reduces the number of programming languages involved, making it easier to focus on the core logic.
- Command-line complexity: Pipes in the shell can be challenging to build, especially with multiple processes.
In conclusion, using subprocess.Popen to pipe multiple processes can be achieved either by delegating the pipeline to the shell or by rewriting the script in Python to eliminate the need for pipes. The latter approach is often preferable due to its simplicity and efficiency.
The above is the detailed content of How to Efficiently Chain Processes Using Python's `subprocess.Popen` with or without Pipes?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










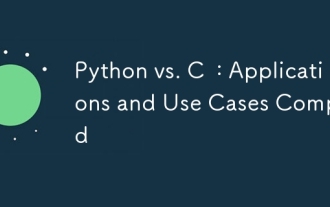
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
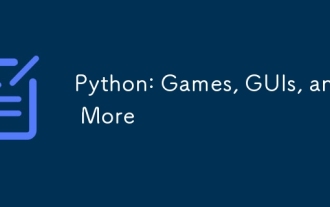
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
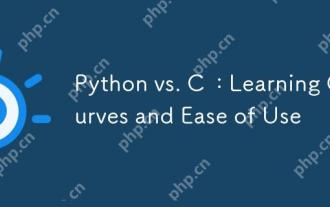
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
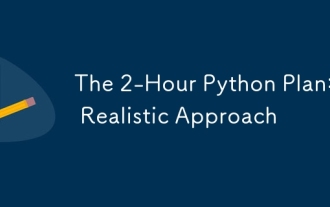
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
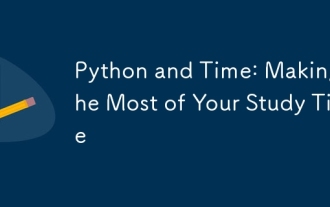
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
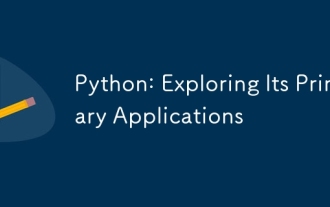
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
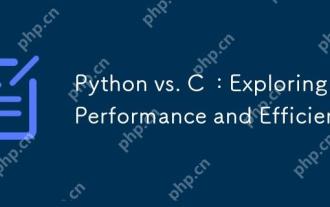
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
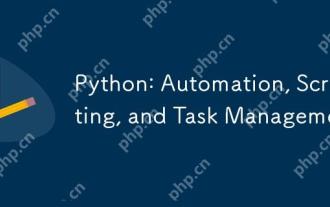
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
