How to Safely Create a Go Slice or Array from an `unsafe.Pointer`?
How to create an array or a slice from an array unsafe.Pointer in Go?
Question:
Given an unsafe pointer to an array, how can we create an array or a slice from it without incurring the cost of memory copy?
Answer:
Creating a Slice Using Reflect:
The recommended approach is to use the reflect package to create a slice header that points to the same underlying data as the unsafe pointer.
// Create a slice header sh := &reflect.SliceHeader{ Data: p, // Unsafe pointer to the array Len: size, Cap: size, } // Use the slice header to create a slice data := *(*[]byte)(unsafe.Pointer(sh))
Creating an Array Using Reflect:
To create an array (pointer to elements in contiguous memory) from an unsafe pointer, we can first create a slice and then take its address:
// Create a slice data := *(*[]byte)(unsafe.Pointer(&reflect.SliceHeader{ Data: p, Len: size, Cap: size, })) // Get the address of the slice (pointer to the first element) arr := (*[size]byte)(unsafe.Pointer(&data[0]))
Caveats and Warnings:
- Ensure that the lifetime of the original array is managed to prevent unexpected memory access.
- Avoid using uintptr variables as references to objects, as garbage collection may occur unexpectedly.
- Use runtime.KeepAlive() if necessary to prevent the original array from being garbage collected before it is referenced by the slice or array created from the unsafe pointer.
The above is the detailed content of How to Safely Create a Go Slice or Array from an `unsafe.Pointer`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










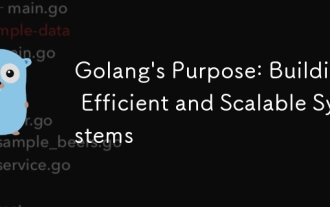
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
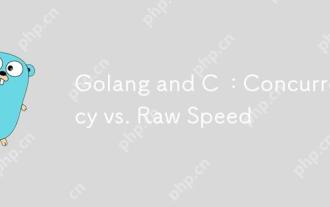
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
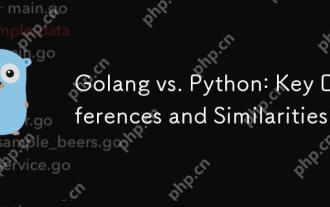
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
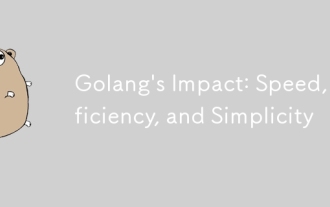
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
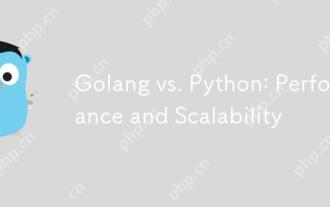
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
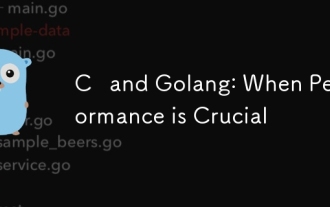
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
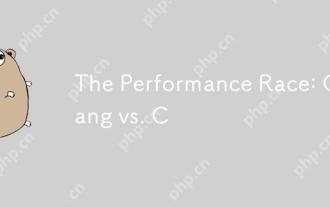
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
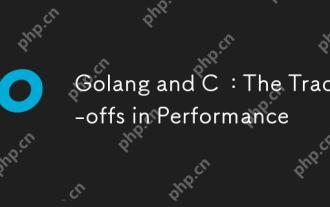
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
