


How Can I Properly Signal the End of Traversal in a Go Binary Tree Equivalence Exercise?
Go Tour Exercise #7: Equivalence of Binary Trees
When attempting the binary trees equivalence exercise in the Go tour, you may encounter a challenge in signaling when there are no more elements left in the trees. The given code attempts to use a channel to communicate values from the trees, but it fails to address this signaling issue.
The Problem
Closing a channel during recursive traversal prematurely terminates the transmission of values. Using close(ch) within the Walk() function closes the channel before all values are sent.
A Solution Using Closure
A closure allows you to create an anonymous function that captures variables from the surrounding scope. This can be used to generate a custom walk function that automatically closes the channel when its execution is complete.
func Walk(t *tree.Tree, ch chan int) { defer close(ch) // Automatically closes the channel when this function returns var walk func(t *tree.Tree) walk = func(t *tree.Tree) { if t == nil { return } walk(t.Left) ch <- t.Value walk(t.Right) } walk(t) }
In this solution, the Walk() function returns a walk closure that handles the traversal of the tree. When the closure exits, it automatically closes the channel, indicating that there are no more values to be sent. This ensures that the receiving end can determine when the traversal is complete.
The above is the detailed content of How Can I Properly Signal the End of Traversal in a Go Binary Tree Equivalence Exercise?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










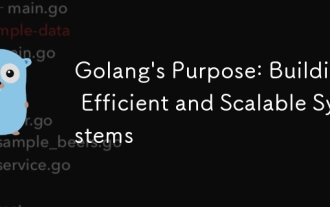
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
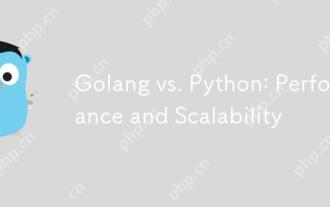
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
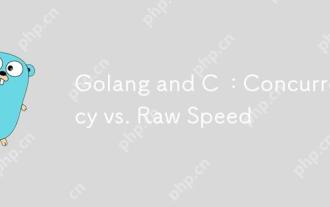
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
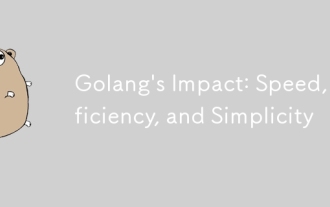
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
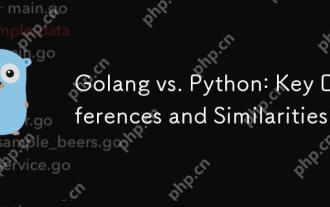
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
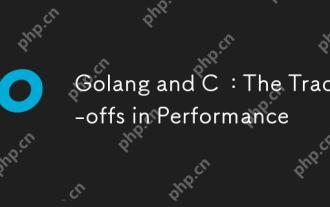
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
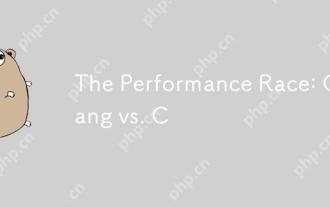
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
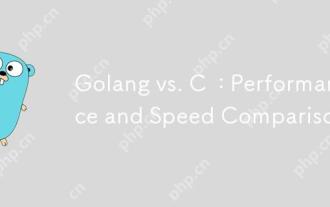
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
