


How Can I Truncate PHP Strings to the Nearest Word Without Mid-Word Cuts?
Truncating PHP Strings to the Nearest Word
Truncating a string to a specific character limit can result in an unsightly cutoff mid-word. To cater to this need, we explore a PHP code snippet that accurately truncates text at the end of the closest word.
Solution 1: Using Wordwrap
The wordwrap function can split text into lines based on the specified maximum width, breaking at word boundaries. To achieve the desired truncation, we can simply utilize the first line from the split text:
$truncated = substr($string, 0, strpos(wordwrap($string, $limit), "\n"));
Solution 2: Token-Based Truncation
However, solution 1 has a limitation: it prematurely cuts the text if a newline character appears before the cutoff point. To mitigate this, we can implement token-based truncation:
function tokenTruncate($string, $limit) { $tokens = preg_split('/([\s\n\r]+)/u', $string, null, PREG_SPLIT_DELIM_CAPTURE); $length = 0; $lastToken = 0; while ($length <= $limit && $lastToken < count($tokens)) { $length += strlen($tokens[$lastToken]); $lastToken++; } return implode(array_slice($tokens, 0, $lastToken)); }
Conclusion
By implementing these solutions, you can effectively truncate PHP strings at the nearest word, ensuring a clean and meaningful display.
The above is the detailed content of How Can I Truncate PHP Strings to the Nearest Word Without Mid-Word Cuts?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


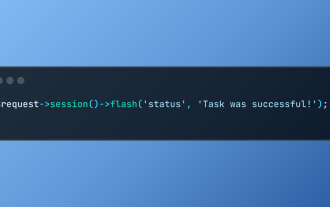
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
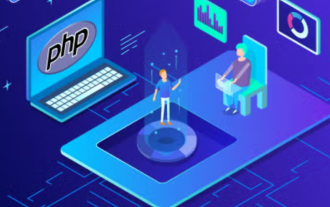
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
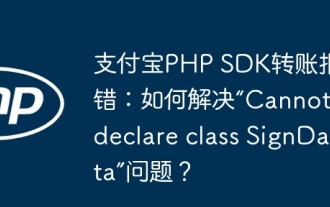
Alipay PHP...
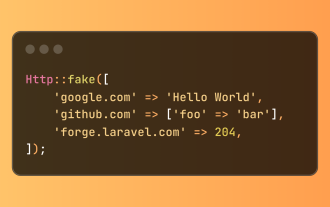
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
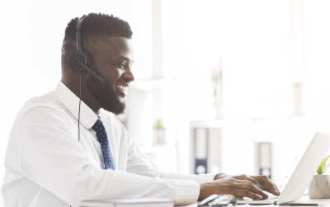
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
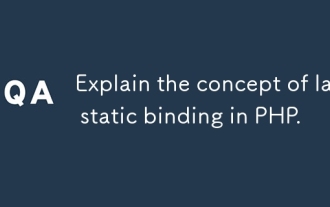
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
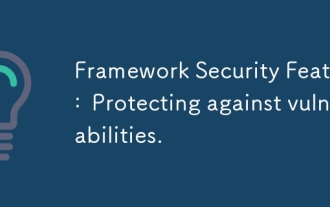
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
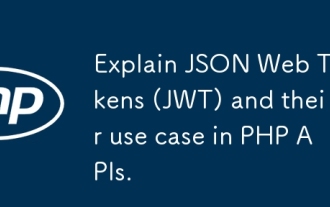
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
