


How to Redirect Students, Teachers, and Admins to Their Respective Activities After Firebase Authentication?
Implementing Multi-User Redirection in a Firebase Voting App
In the context of a Firebase-based voting application, you are wondering how to redirect three distinct types of users (STUDENTS, TEACHERS, and ADMINS) to their respective activities after login. Here's a modified version of your code to cater to this requirement:
mAuthListener = new FirebaseAuth.AuthStateListener() { @Override public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) { FirebaseUser firebaseUser = FirebaseAuth.getInstance().getCurrentUser(); if (firebaseUser != null) { String uid = firebaseUser.getUid(); DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference(); DatabaseReference usersRef = rootRef.child("users").child(uid); ValueEventListener userListener = new ValueEventListener() { @Override public void onDataChange(@NonNull DataSnapshot dataSnapshot) { // Assert that the DataSnapshot is valid if (!dataSnapshot.exists()) { Log.e(TAG, "Error: DataSnapshot not found"); return; } // Check the user type and redirect accordingly if (dataSnapshot.child("type").getValue(Long.class) == 1) { startActivity(new Intent(MainActivity.this, student.class)); } else if (dataSnapshot.child("type").getValue(Long.class) == 2) { startActivity(new Intent(MainActivity.this, teacher.class)); } else if (dataSnapshot.child("type").getValue(Long.class) == 3) { startActivity(new Intent(MainActivity.this, admin.class)); } } @Override public void onCancelled(@NonNull DatabaseError databaseError) { Log.e(TAG, databaseError.getMessage()); } }; usersRef.addListenerForSingleValueEvent(userListener); } } };
Key Changes:
- The uidRef has been updated to point to the users node under the Firebase root.
- Instead of checking only for the existence of a student, the code now checks the type child node to determine the user's type.
- Three separate conditionals have been added to handle all three user types.
The above is the detailed content of How to Redirect Students, Teachers, and Admins to Their Respective Activities After Firebase Authentication?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










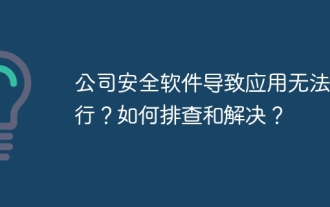
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
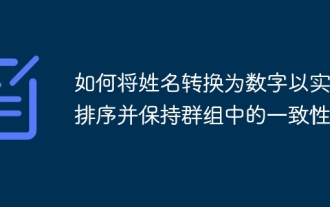
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
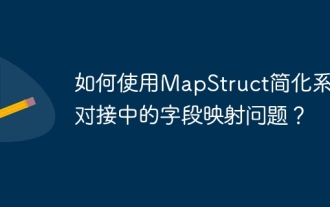
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
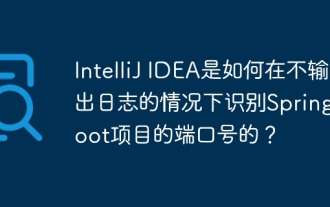
Start Spring using IntelliJIDEAUltimate version...
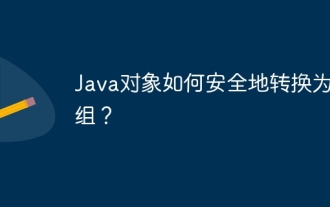
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
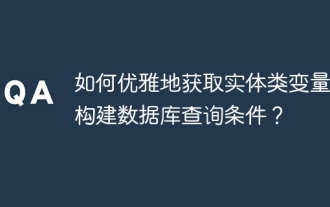
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
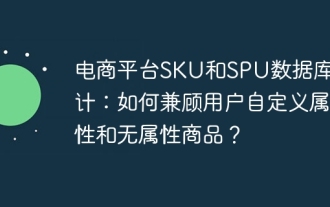
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
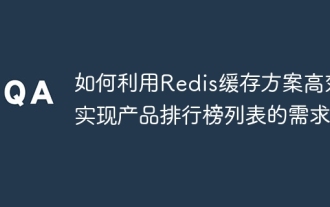
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
