


How Can I Truncate Strings in PHP While Preserving Word Boundaries?
Maintaining Semantic Integrity: Truncating Strings at the Closest Word Boundary
When dealing with strings in programming, it's often necessary to truncate them to fit a specific length. However, naively chopping off characters can lead to awkward or incorrect results, especially if the truncation occurs mid-word.
In PHP, we have a few options for truncating strings while preserving semantic integrity.
Using Wordwrap and Substring
The wordwrap function can split a string into multiple lines, respecting word boundaries. By specifying a maximum width, we can create a line break at the closest word before the desired length. The following code snippet demonstrates this approach:
$string = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua."; $desired_width = 200; $truncated_string = substr($string, 0, strpos(wordwrap($string, $desired_width), "\n"));
Now, $truncated_string contains the desired text, but only up to the end of the last word before the 200th character.
Handling Edge Cases
This approach works well, but it doesn't handle the case where the original string is shorter than the desired width. To address this, we can wrap the logic in a conditional statement:
if (strlen($string) > $desired_width) { $truncated_string = substr($string, 0, strpos(wordwrap($string, $desired_width), "\n")); }
Dealing with Newlines
A subtle issue arises when the string contains a newline character before the desired truncation point. In such cases, the wordwrap function may create a line break prematurely. To overcome this, we can use a more sophisticated regular expression-based approach:
function tokenTruncate($string, $desired_width) { $parts = preg_split('/([\s\n\r]+)/u', $string, null, PREG_SPLIT_DELIM_CAPTURE); $parts_count = count($parts); $length = 0; $last_part = 0; for (; $last_part < $parts_count; ++$last_part) { $length += strlen($parts[$last_part]); if ($length > $desired_width) { break; } } return implode(array_slice($parts, 0, $last_part)); }
This function iterates over word tokens and stops when the total length exceeds the desired width. It then rebuilds the truncated string, ensuring that it ends at a word boundary.
Testing and Handling Complexities
Unit testing is crucial to validate the functionality of our code. The provided PHP PHPUnit test class demonstrates the correct behavior of the tokenTruncate function.
Special UTF8 characters like 'à' may require additional handling. This can be achieved by adding 'u' to the end of the regular expression:
$parts = preg_split('/([\s\n\r]+)/u', $string, null, PREG_SPLIT_DELIM_CAPTURE);
By employing these techniques, we can confidently truncate strings in PHP, maintaining their semantic integrity and ensuring aesthetically pleasing and consistent results.
The above is the detailed content of How Can I Truncate Strings in PHP While Preserving Word Boundaries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










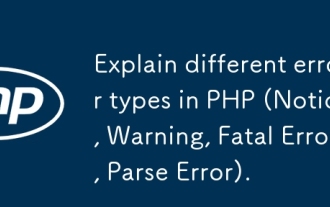
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
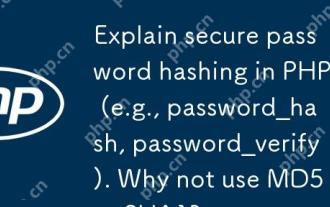
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
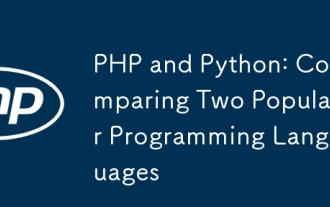
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
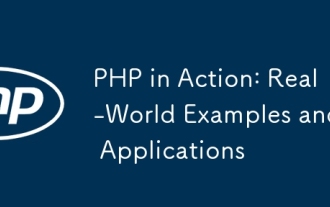
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
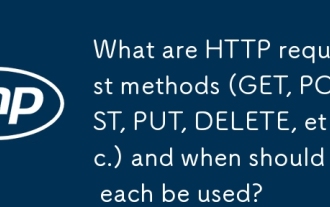
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
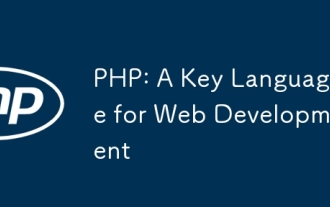
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
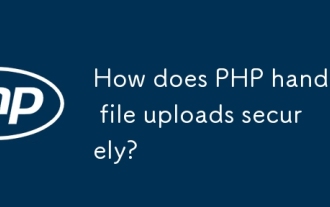
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
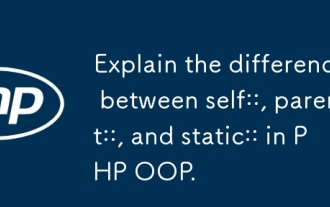
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
