How Can I Handle Checked Exceptions Thrown from Java 8 Lambdas and Streams?
Throwing Checked Exceptions from Java 8 Lambdas and Streams
Unlike runtime exceptions, checked exceptions require explicit handling in Java code. However, when using lambda expressions and streams, developers may encounter challenges throwing checked exceptions. This article explores the limitations and potential solutions for handling checked exceptions in these contexts.
The Limitation:
Current Java 8 syntax does not provide a direct mechanism to throw checked exceptions from within lambda expressions used in streams. The following code snippet demonstrates the compilation error encountered:
import java.util.List; import java.util.stream.Stream; public class CheckedStream { public List<Class> getClasses() throws ClassNotFoundException { Stream.of("java.lang.Object", "java.lang.Integer", "java.lang.String") .map(className -> Class.forName(className)) .collect(Collectors.toList()); } }
The issue stems from the fact that the functional interfaces used in streams, such as Function and Stream, do not declare type parameters to support throwing checked exceptions. Consequently, the compiler cannot infer the precise exception type and reports a compilation error.
The Oracle Dilemma:
The omission of this functionality is attributed to an oversight during the design of Java 8's functional interfaces. The Java community has widely criticized Oracle for this limitation, with many arguing that it is a significant bug in the API and a drawback of checked exceptions in general.
Alternatives and Workarounds:
While Java 8 does not directly support throwing checked exceptions from lambdas, there are workarounds available:
1. Wrapping Checked Exceptions in Runtime Exceptions:
This approach involves wrapping checked exceptions in runtime exceptions and then throwing the wrapped exceptions within lambda expressions.
// Import the necessary class. import java.io.IOException; // Create a wrapper class to wrap checked exceptions. public class CheckedExceptionWrapper { public static void main(String[] args) { // Create a stream of strings. Stream<String> stream = Stream.of("file1.txt", "file2.txt", "file3.txt"); // Map the stream using a lambda that wraps checked exceptions. stream = stream.map(file -> { try { // Read the file. FileReader reader = new FileReader(file); reader.close(); return file; } catch (IOException e) { // Wrap the checked exception in a runtime exception. throw new RuntimeException(e); } }); // Collect the results. List<String> files = stream.collect(Collectors.toList()); } }
2. Using Checked Supplier:
The CheckedSupplier interface from the guava library allows you to create suppliers that throw checked exceptions. You can use this interface to wrap checked exception-throwing code in lambda expressions.
// Import the necessary class. import com.google.common.base.CheckedSupplier; // Create a checked supplier that throws a checked exception. CheckedSupplier<String> supplier = () -> { // Code that throws a checked exception. throw new RuntimeException(); }; // Get the result from the supplier. try { String result = supplier.get(); } catch (Exception e) { // Handle the exception. }
3. Rewriting Code to Avoid Using Checked Exceptions:
Instead of using checked exceptions, consider rewriting your code to handle errors in a different way. For example, you could use the Optional class to represent optional values or throw unchecked exceptions and handle them using try-catch blocks.
4. Using Catch and Throw Blocks:
This approach is straightforward but can make your code verbose and difficult to read.
// Example for Java 7 with try/catch try { // Code that throws a checked exception. throw new RuntimeException(); } catch (Exception e) { // Handle the exception. }
Conclusion:
While Java 8's syntax does not directly support throwing checked exceptions from lambdas and streams, there are workarounds available. Consider carefully which approach is appropriate for your specific situation.
The above is the detailed content of How Can I Handle Checked Exceptions Thrown from Java 8 Lambdas and Streams?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










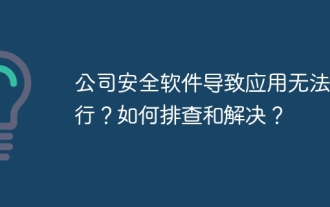
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
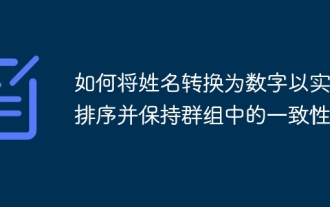
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
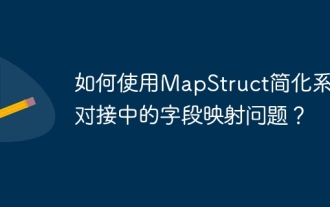
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
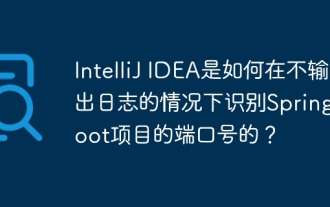
Start Spring using IntelliJIDEAUltimate version...
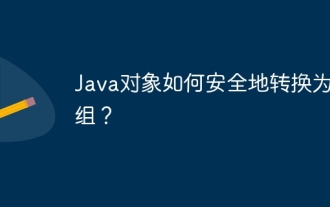
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
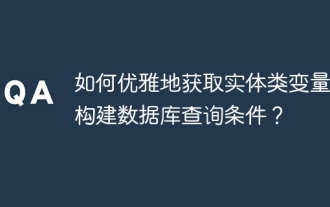
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
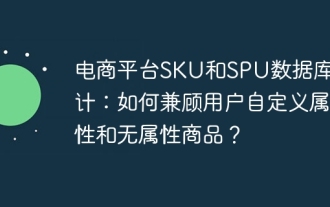
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
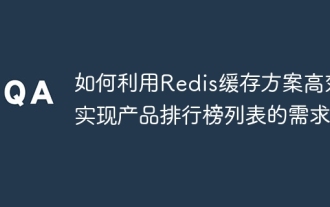
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
