How Can I Truly Implement Non-Blocking Functions in Node.js?
How to Properly Implement Non-Blocking Functions in Node.js
Despite its asynchronous nature, Node.js doesn't provide a straightforward way to execute code non-blockingly. Functions wrapped in Promises, as you did in your example, still execute synchronously and block the main thread.
Understanding Node.js Execution Model
Unlike true asynchronous languages, Node.js runs JavaScript code in a single-threaded event loop. All code, including Promise executor functions, blocks the main thread until completion. Therefore, the expectation of non-blocking behavior in your code is incorrect.
Achieving Actual Non-Blocking Code
To create genuinely non-blocking code, you must employ techniques that remove intensive tasks from the main thread:
- Child Processes: Create separate child processes to execute time-consuming operations. When completed, you'll be notified asynchronously.
- Worker Threads: Leverage the experimental Worker Threads feature in Node.js v11 to run tasks in separate threads.
- Native Code Add-ons: Write your own native code extensions using libuv threads or OS-level asynchronous tools.
- Asynchronous APIs: Utilize existing asynchronous APIs, such as file I/O or database queries, to avoid lengthy tasks in the main thread.
Example: Remedying Your Code
To exhibit non-blocking behavior in your code, incorporate a setTimeout() function. However, this only alters the timing of code execution, not its blocking nature:
function longRunningFunc(val, mod) { return new Promise((resolve, reject) => { setTimeout(() => { // Time-consuming loop resolve(sum) }, 10); }) }
Conclusion
While Promises are valuable for handling asynchronous tasks, they alone do not make functions non-blocking. To achieve true non-blocking behavior, consider the techniques outlined above to execute code outside the main thread. By understanding the limitations of Node.js's execution model, you can develop code that utilizes its asynchronous capabilities effectively.
The above is the detailed content of How Can I Truly Implement Non-Blocking Functions in Node.js?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










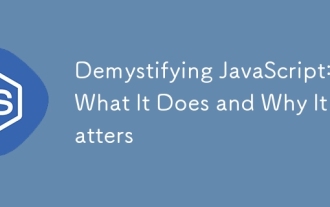
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
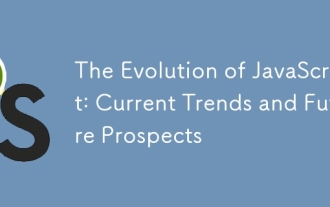
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
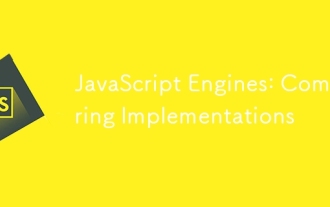
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
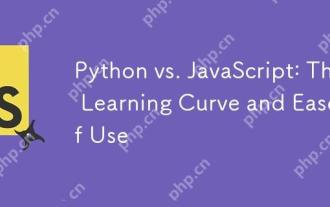
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
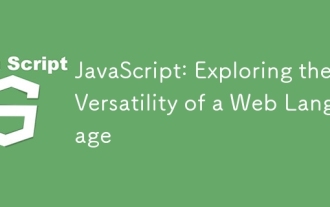
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
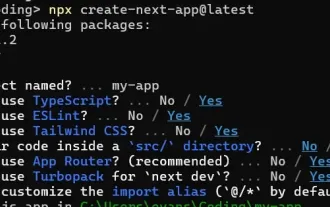
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
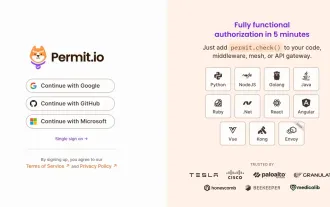
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
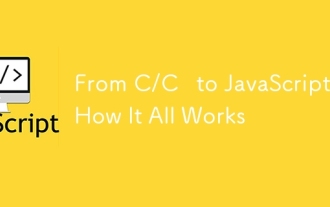
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
