


How Can I Get the Exact CSS Property Value (Including Units) of an Element in JavaScript?
Get Element CSS Property Value as Set (Percent/Em/Px)
Obtaining the CSS property value of an element as specified in the style rules can be challenging, especially when the property has been set in various units. In Google Chrome, the getComputedStyle() method and jQuery's css() method both return the current value in pixels.
Solution: getMatchedStyle Function
To address this issue, a JavaScript function called getMatchedStyle() was developed. It takes an element and a property as arguments and returns the value as it was set in the CSS rules.
function getMatchedStyle(elem, property) { // Check element's own style first var val = elem.style.getPropertyValue(property); if (elem.style.getPropertyPriority(property)) return val; // Get matched CSS rules var rules = getMatchedCSSRules(elem); // Iterate rules in reverse order of priority for (var i = rules.length; i--;) { var r = rules[i]; var important = r.style.getPropertyPriority(property); // Reset value if important or not yet set if (val == null || important) { val = r.style.getPropertyValue(property); if (important) break; } } return val; }
Example
Consider the following HTML code with CSS rules:
<div class="b">div 1</div> <div>
The following JavaScript code uses getMatchedStyle() to obtain the width values for the divs:
var d = document.querySelectorAll('div'); for (var i = 0; i < d.length; ++i) { console.log("div " + (i + 1) + ": " + getMatchedStyle(d[i], 'width')); }
Results:
div 1: 100px div 2: 50% div 3: auto div 4: 44em
The above is the detailed content of How Can I Get the Exact CSS Property Value (Including Units) of an Element in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










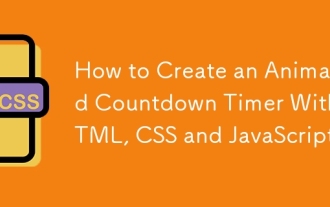
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
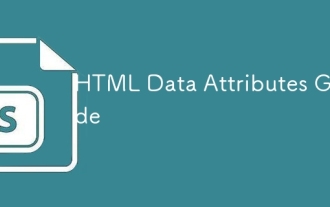
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
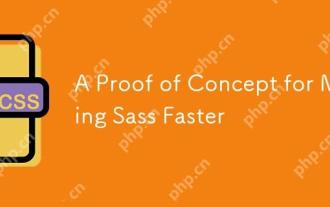
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
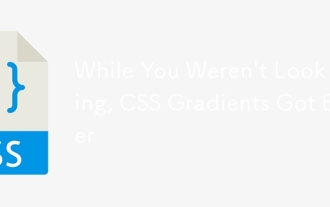
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
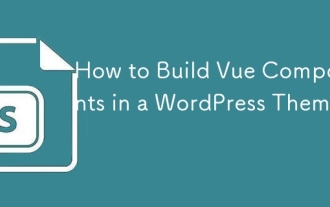
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
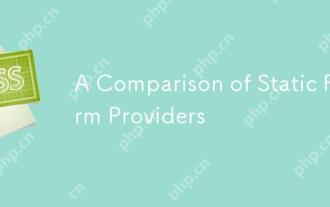
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
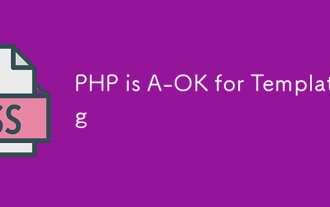
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
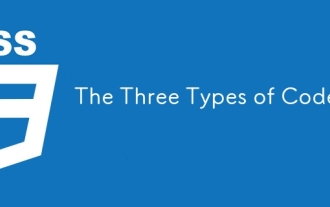
Every time I start a new project, I organize the code I’m looking at into three types, or categories if you like. And I think these types can be applied to
