


How to Effectively Map Composite Keys in JPA and Hibernate using `@EmbeddedId` and `@IdClass`?
Mapping Composite Keys with JPA and Hibernate
When mapping entities to database tables, it's common to use primary keys to uniquely identify records. Composite keys, consisting of multiple columns, are often used in legacy database systems. Both JPA and Hibernate provide annotations to handle composite keys effectively.
Using EmbeddedId
The EmbeddedId annotation denotes that a Java class represents a composite primary key. It maps the class to the table's clustered primary key. The class must:
- Be annotated with @Embeddable
- Define public or protected properties corresponding to the primary key columns
- Have a public constructor that takes values for all primary key columns
- Implement equals() and hashCode() methods for value equality
Example with EmbeddedId
@Entity public class Time { @EmbeddedId private TimePK timePK; private String src; private String dst; private Integer distance; private Integer price; } @Embeddable public class TimePK { private Integer levelStation; private Integer confPathID; // Constructor, equals(), and hashCode() methods omitted for brevity }
Using IdClass
The IdClass annotation specifies that multiple fields or properties of an entity class make up a composite primary key. The class must:
- Extend a serializable object (e.g., java.io.Serializable)
- Have a public no-argument constructor
- Define public (or protected, with property-based access) fields or properties corresponding to the primary key columns
- Implement equals() and hashCode() methods for value equality
Example with IdClass
@Entity @IdClass(TimePK.class) public class Time { @Id private Integer levelStation; @Id private Integer confPathID; private String src; private String dst; private Integer distance; private Integer price; } public class TimePK implements Serializable { private Integer levelStation; private Integer confPathID; // Constructor, equals(), and hashCode() methods omitted for brevity }
Choosing Between EmbeddedId and IdClass
EmbeddedId creates a separate class for the composite key, while IdClass uses the entity class itself to hold the primary key fields. EmbeddedId is more explicit and suggests a meaningful entity, while IdClass is better for combinations of fields that don't carry an independent meaning.
References
-
JPA 1.0 specification: https://jcp.org/en/jsr/detail?id=220
- Section 2.1.4: https://jspec.dev.java.net/nonav/javadoc/javax/persistence/doc-files/javax/persistence/spec/version-1_0-final.doc.html#section_2.1.4
- Section 9.1.14: https://jspec.dev.java.net/nonav/javadoc/javax/persistence/doc-files/javax/persistence/spec/version-1_0-final.doc.html#section_9.1.14
- Section 9.1.15: https://jspec.dev.java.net/nonav/javadoc/javax/persistence/doc-files/javax/persistence/spec/version-1_0-final.doc.html#section_9.1.15
The above is the detailed content of How to Effectively Map Composite Keys in JPA and Hibernate using `@EmbeddedId` and `@IdClass`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


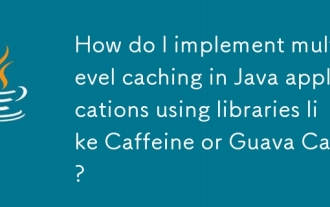
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
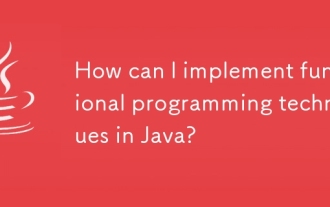
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
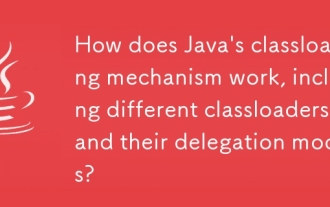
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
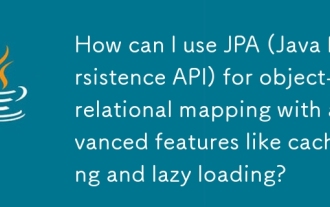
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
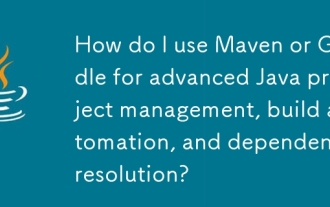
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
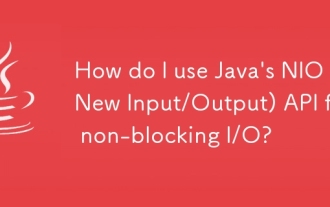
This article explains Java's NIO API for non-blocking I/O, using Selectors and Channels to handle multiple connections efficiently with a single thread. It details the process, benefits (scalability, performance), and potential pitfalls (complexity,
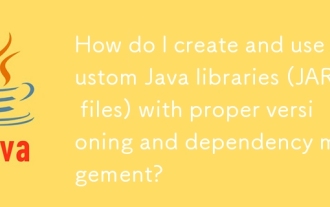
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
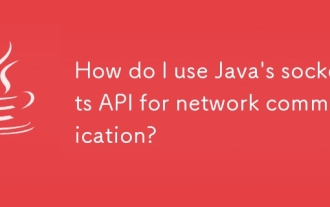
This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
