


How Do I Properly Escape Backslashes in Python String Literals to Avoid Decoding Errors?
Dec 11, 2024 pm 03:22 PMEscaping a Backslash in a Python String Literal
When trying to include an actual backslash in a Python string literal (without using it as an escape sequence), an error might occur indicating a decoding issue. To resolve this, prefix the string with the letter 'r'.
For instance, consider the following code:
import os path = os.getcwd() final = path +'\xulrunner.exe ' + path + '\application.ini' print(final)
This code will result in an error because the backslashes are interpreted as escape sequences. To fix this, prefix the strings with 'r':
final= path + r'\xulrunner.exe ' + path + r'\application.ini'
Now, the output will be as expected:
C:\Users\me\xulrunner.exe C:\Users\me\application.ini
However, it's generally better practice to use os.path.join for constructing file paths, as it automatically handles the platform-specific path separator. The following code uses os.path.join to create the same path:
final = (os.path.join(path, 'xulrunner.exe') + ' ' + os.path.join(path, 'application.ini'))
Additionally, note that using forward slashes (/) in file paths is acceptable, as they will be automatically converted to the appropriate separator for the operating system.
The above is the detailed content of How Do I Properly Escape Backslashes in Python String Literals to Avoid Decoding Errors?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
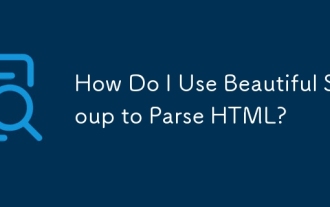
How Do I Use Beautiful Soup to Parse HTML?
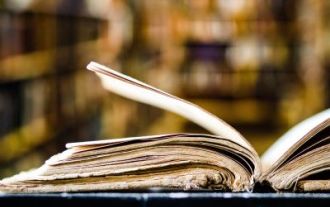
How to Use Python to Find the Zipf Distribution of a Text File
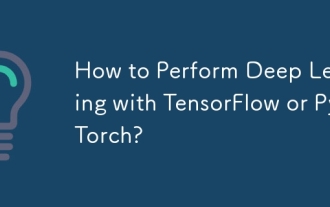
How to Perform Deep Learning with TensorFlow or PyTorch?
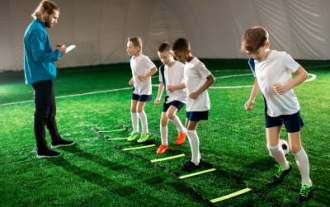
Introduction to Parallel and Concurrent Programming in Python
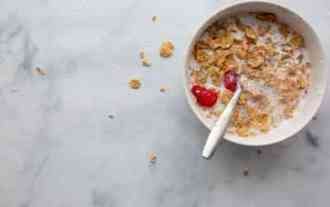
Serialization and Deserialization of Python Objects: Part 1
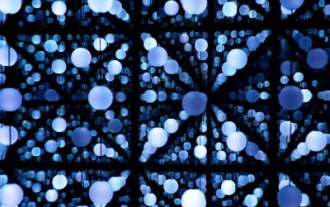
How to Implement Your Own Data Structure in Python
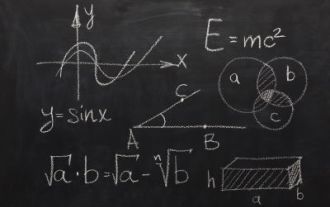
Mathematical Modules in Python: Statistics
