How Can I Secure MySQL Queries in Python Against SQL Injection?
Securing MySQL Queries in Python to Prevent SQL Injection
When querying MySQL databases from Python, preventing SQL injection attacks is crucial. This involves ensuring that user-provided variables don't interfere with the query structure.
Execute parameterized queries using the "execute" function with %s as placeholders for variables. Pass the variables as a list or tuple as the second parameter to execute.
c = db.cursor() max_price = 5 c.execute("SELECT spam, eggs, sausage FROM breakfast WHERE price < %s", (max_price,))
Avoid direct string substitution using %. Instead, use a comma as the placeholder:
c.execute("SELECT spam, eggs, sausage FROM breakfast WHERE price < %s", (max_price,))
Do not enclose the placeholder with single quotes if the parameter is a string:
c.execute("SELECT spam, eggs, sausage FROM breakfast WHERE price < %s", (max_price,))
By following these best practices, you can reduce the risk of SQL injection attacks and ensure the security of your MySQL connections in Python.
The above is the detailed content of How Can I Secure MySQL Queries in Python Against SQL Injection?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


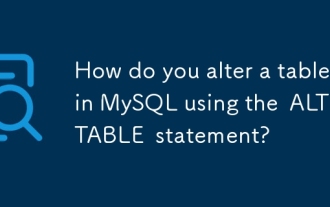
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
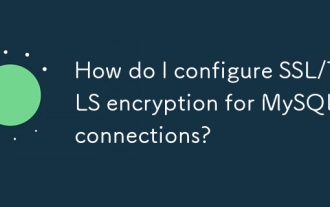
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
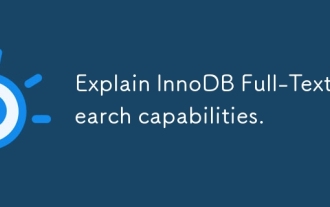
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
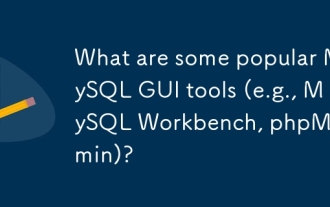
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
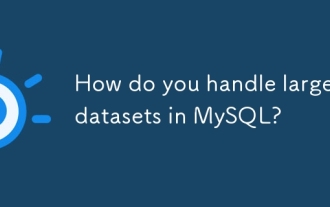
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
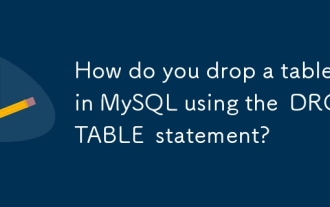
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
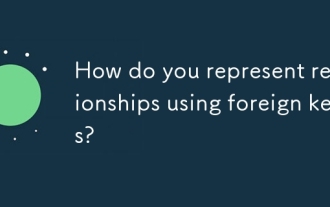
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
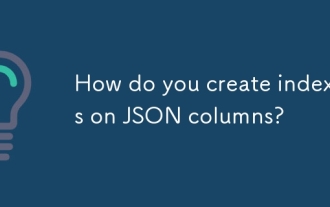
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
