Call vs. Apply in JavaScript: When Should I Use Which?
Call vs. Apply: Unveiling the Differences in Function Invocation
In JavaScript, invoking a function can be accomplished through various methods, with Function.prototype.apply() and Function.prototype.call() being two prominent choices. However, these methods exhibit key differences that can influence their suitability in different scenarios.
Functional Similarity
Both call and apply share a common purpose: they facilitate the invocation of a function. However, the manner in which they handle arguments differs significantly.
apply (ValueForThis, ArrayOfArgs)
The apply() method requires two arguments: the value to be bound to the "this" keyword within the function, and an array containing the arguments that the function should receive. The apply() method expands the array into individual arguments before passing them to the function.
call (ValueForThis, Arg1, Arg2, ...)
In contrast, the call() method allows the arguments to be passed as distinct parameters, similar to how regular function calls are made. The value bound to the "this" keyword is specified as the first argument, followed by the remaining arguments that the function requires.
Performance Considerations
Benchmarking reveals that call is marginally faster than apply in most scenarios. This is because call operates with a slightly lower overhead, since it does not require the additional processing involved in expanding the arguments.
Practical Considerations
The distinction between call and apply lies primarily in their suitability for handling different types of arguments.
- Use call when passing a fixed number of arguments explicitly.
- Consider apply when invoking a function with a variable number of arguments or when you need to pass an array as an argument.
Example Usage
The provided code snippet demonstrates the usage of both call and apply:
function theFunction(name, profession) { console.log("My name is " + name + " and I am a " + profession +"."); } theFunction("John", "fireman"); theFunction.apply(undefined, ["Susan", "school teacher"]); theFunction.call(undefined, "Claude", "mathematician"); theFunction.call(undefined, ...["Matthew", "physicist"]); // using the spread operator
Understanding the nuances of call and apply empowers developers to invoke functions effectively, tailoring their approach based on the nature of the arguments and performance considerations.
The above is the detailed content of Call vs. Apply in JavaScript: When Should I Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


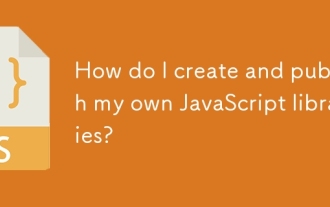
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
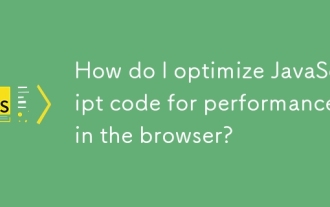
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
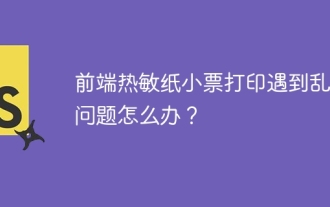
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
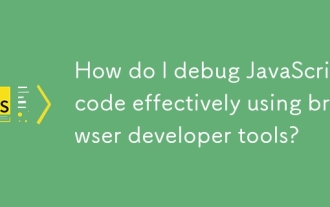
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
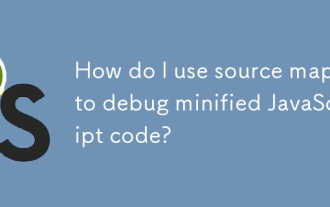
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
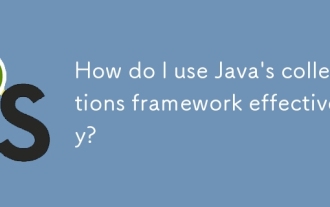
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
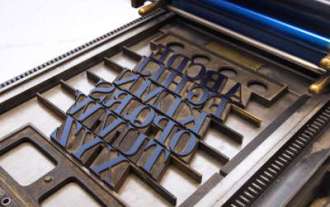
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
