When Should You Use Java Enums and What Are Their Advantages?
Enums: Understanding Their Value and Applications
Introduction:
Enums, short for enumerated types, are powerful constructs in Java that enable developers to define a set of constants with specific names and values. Their introduction and evolution in recent Java versions have significantly expanded their capabilities, making them invaluable tools in everyday programming.
When to Use Enums:
The primary purpose of enums is to represent variables that can only accept a finite set of predefined values. Prime examples include status indicators (such as "Active" or "Terminated") or flag options (e.g., "Enabled" or "Disabled").
Benefits of Enums:
Using enums offers several advantages over using integers or strings to represent these constants:
- Compile-time Checking: Enums enforce type safety at compile time, preventing invalid values from being assigned. This enhances code robustness and reduces the likelihood of runtime errors.
- Improved Documentation: Enum constants provide explicit and meaningful names, making it easier to understand the intended usage and potential values.
- Prevention of Overuse: Overuse of enums can highlight potential design issues, suggesting that methods may have太多的responsibility. Enums encourage the separation of distinct operations into different methods.
Example:
Consider the following example of a method that counts foobangs based on their type:
Using Integers:
public int countFoobangs(int type) { switch (type) { case 1: return countGreenFoobangs(); case 2: return countWrinkledFoobangs(); case 3: return countSweetFoobangs(); default: return countAllFoobangs(); } }
Using Enums:
public enum FB_TYPE {GREEN, WRINKLED, SWEET, ALL} public int countFoobangs(FB_TYPE type) { switch (type) { case GREEN: return countGreenFoobangs(); case WRINKLED: return countWrinkledFoobangs(); case SWEET: return countSweetFoobangs(); case ALL: return countAllFoobangs(); } }
By using enums, the code becomes more concise, readable, and less prone to errors. The compiler ensures that only valid type values can be passed to the countFoobangs method. Additionally, the usage of enums is self-documenting, clarifying the intended purpose of the different types.
The above is the detailed content of When Should You Use Java Enums and What Are Their Advantages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










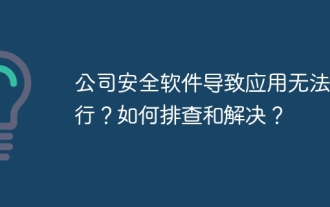
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
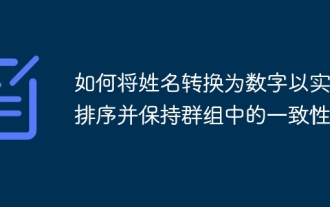
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
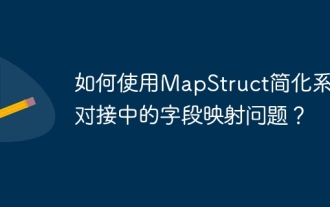
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
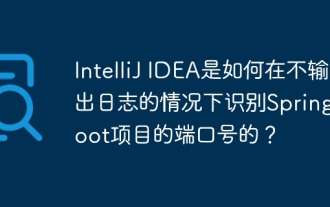
Start Spring using IntelliJIDEAUltimate version...
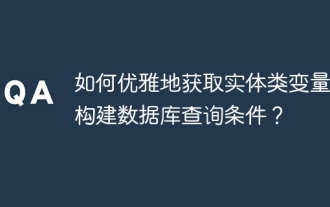
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
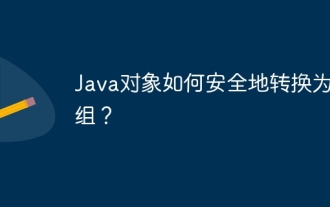
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
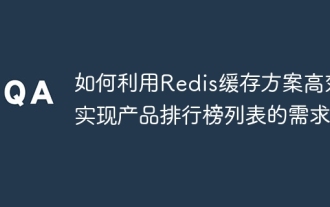
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
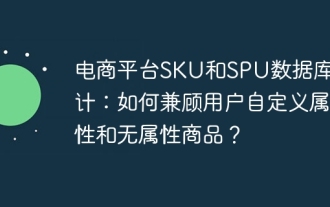
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
