When Does a Go Variable Become Unreachable, Even Within Its Scope?
When Does a Variable Become Unreachable in Go?
In Go, a variable becomes unreachable when the runtime determines that it is impossible for the program to reference it again. This can occur even if the variable has not yet left its declared scope.
Understanding the Example
Consider the example you provided:
type File struct { d int } d, err := syscall.Open("/file/path", syscall.O_RDONLY, 0) p := &FILE{d} runtime.SetFinalizer(p, func(p *File) { syscall.Close(p.d) })
The p variable points to a struct containing a file descriptor. A finalizer is attached to p to close the file descriptor when p becomes unreachable. However, the last use of p in Go code is when it is passed to syscall.Read().
Why p Becomes Unreachable
The implementation of syscall.Read() may access the file descriptor after the call is initiated. This means that p is used outside of the Go code, which allows the runtime to mark it as unreachable. Even though p has not yet left its scope, it is no longer live within the Go codebase.
Purpose of runtime.KeepAlive()
To prevent this premature marking of p as unreachable, you can use runtime.KeepAlive() as demonstrated in the example:
runtime.KeepAlive(p)
By referencing p in this function call, the runtime is instructed to keep it alive until syscall.Read() returns. This ensures that the file descriptor remains valid for the duration of the syscall.
Conclusion
In Go, a variable can become unreachable when it is no longer referenced in the program's code and is being used externally, even if it has not yet left its declared scope. runtime.KeepAlive() provides a clear and effective way to prevent variables from becoming unreachable prematurely, ensuring the proper execution of finalizers and avoiding unexpected behavior.
The above is the detailed content of When Does a Go Variable Become Unreachable, Even Within Its Scope?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










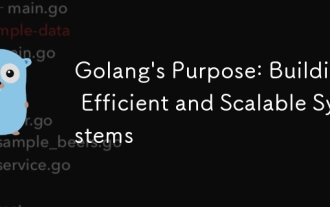
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
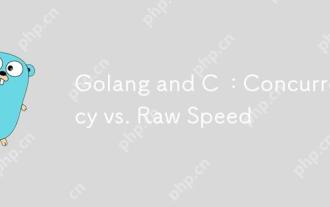
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
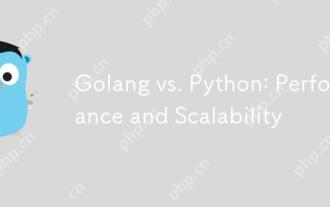
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
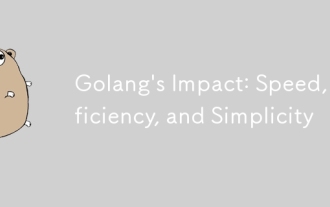
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
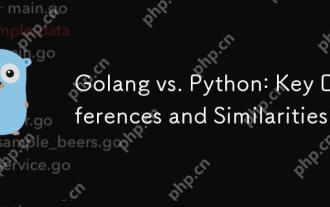
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
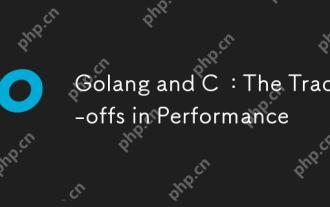
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
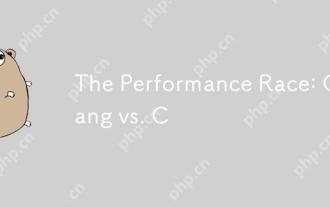
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
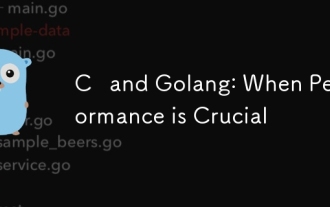
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
