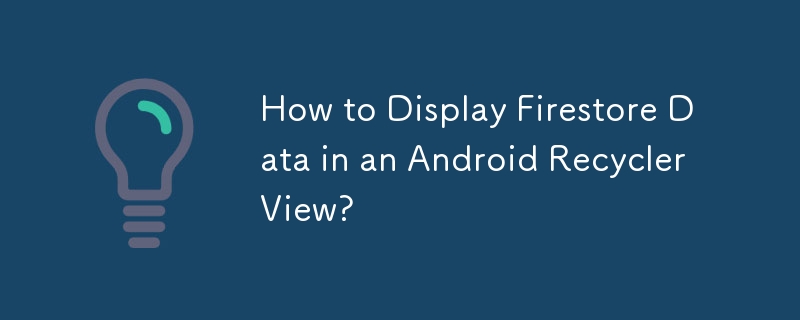
Displaying Firestore Data in a RecyclerView with Android
One of the primary tasks when developing Android applications that interact with a database is efficiently displaying the data in a user-friendly format. Among the various UI elements available, RecyclerView has proven to be a powerful choice for displaying large datasets in a scrollable list.
In this article, we will focus on showcasing how to successfully retrieve data from an existing Firestore database and display it within a RecyclerView in an Android application.
Approach
-
Prepare Your Database: Ensure that you have successfully created a Firestore database with the necessary collections and documents.
-
Create a Model Class: Define a model class that represents the data you want to retrieve from Firestore. This class should encapsulate the data structure of each document in the collection.
-
Retrieve Data from Firestore: Use a Firestore Query to specify which data to retrieve. You can filter, sort, and specify the limit of the data as needed.
-
Create a FirestoreRecyclerAdapter: The FirestoreRecyclerAdapter acts as an adapter between your Firestore Query and the RecyclerView. It manages the data updates and efficiently binds the data to the RecyclerView.
-
Create a RecyclerView.ViewHolder: Define a ViewHolder class that holds the individual views for each row item in the RecyclerView. Connect the views to the data fields in your model class.
-
Bind the Data to the RecyclerView: In the FirestoreRecyclerAdapter, use the onBindViewHolder() method to bind the data from the documents to the views in the ViewHolder.
-
Populate the RecyclerView: Specify the adapter for the RecyclerView and call the startListening() method to start receiving updates from Firestore.
-
Handle Lifecycle Events: Override the onStart() and onStop() methods in your activity or fragment to ensure proper handling of activity lifecycle events and prevent memory leaks.
Example
For a detailed example, let's consider a Firestore database with a collection named "products" containing documents with fields like "productName." We want to display the product names in a RecyclerView.
-
Model Class: Create a ProductModel class that represents the documents in the "products" collection.
-
Firestore Query: Create a Firestore Query to retrieve the product names in ascending order.
-
FirestoreRecyclerAdapter: Initialize a FirestoreRecyclerAdapter with the Query and ProductModel class.
-
ViewHolder Class: Create a ProductViewHolder class that holds a TextView to display the product name.
-
Bind the Data: In the FirestoreRecyclerAdapter's onBindViewHolder(), retrieve the product name from the document and set it in the TextView of the ViewHolder.
-
Populate the RecyclerView: Set the adapter for the RecyclerView and call startListening().
-
Handle Lifecycle Events: Override onStart() and onStop() to manage the adapter's listening behavior.
Conclusion
By following the steps outlined above, you can effectively display data from a Firestore database in a RecyclerView within your Android application. This approach provides an efficient and flexible way to manage and present large datasets from cloud databases in a user-friendly and intuitive manner.
The above is the detailed content of How to Display Firestore Data in an Android RecyclerView?. For more information, please follow other related articles on the PHP Chinese website!