


Synchronous vs. Asynchronous Programming in Node.js: What's the Difference?
Asynchronous vs. Synchronous Programming: A Detailed Examination in Node.js
When working with Node.js, understanding the difference between synchronous and asynchronous programming is crucial. Let's delve into the specifics and explore the key differences between these two approaches.
Synchronous Programming
In synchronous code, the program flow follows a linear path. Each line of code executes sequentially, and the program will not proceed to the next line until the current line has been completed. This means that if a function performs a slow operation, the entire program will be blocked until the operation is finished.
Asynchronous Programming
In contrast to synchronous programming, asynchronous programming allows functions to execute concurrently. When a function initiates a potentially slow operation, such as a database query, it returns control to the main program flow and continues executing other code. Once the operation is complete, the function is "notified" and the program can handle the result. This approach is more efficient as it allows the program to continue executing instead of waiting for the slow operation to finish.
Example
Let's consider two code examples to illustrate the difference:
Synchronous Code:
var result = database.query("SELECT * FROM hugetable"); console.log("Hello World");
In this code, the database.query function will block the program until the query is completed and the result is assigned to the result variable. Only then will the console.log statement be executed.
Asynchronous Code:
database.query("SELECT * FROM hugetable", function(rows) { var result = rows; }); console.log("Hello World");
In this example, the database.query function does not block the program. Instead, it schedules a callback function to be executed once the query is complete. The console.log statement will be executed immediately, demonstrating that the program can continue executing while the query is being processed in the background.
Output
If we run these two code snippets, the output would be:
// Synchronous Query finished Hello World // Asynchronous Hello World Query finished
This shows that in the synchronous example, the program blocks and waits for the query to finish before printing the "Hello World" message. In the asynchronous example, the "Hello World" message is printed immediately, while the query is still being executed in the background.
Benefits of Asynchronous Programming
Asynchronous programming offers several advantages, including:
- Improved performance by allowing concurrent execution of tasks.
- Avoidance of blocking operations that can hinder the responsiveness of the program.
- Support for event-driven programming, where tasks are executed in response to specific events.
Conclusion
Understanding the difference between synchronous and asynchronous programming is fundamental in Node.js development. Asynchronous programming is preferred as it promotes efficient and responsive applications by allowing tasks to be executed concurrently.
The above is the detailed content of Synchronous vs. Asynchronous Programming in Node.js: What's the Difference?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










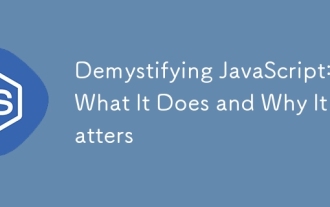
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
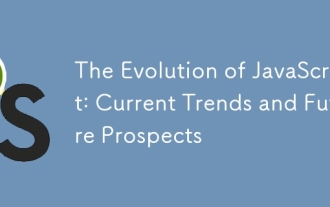
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
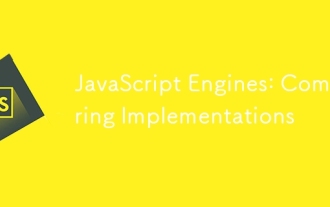
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
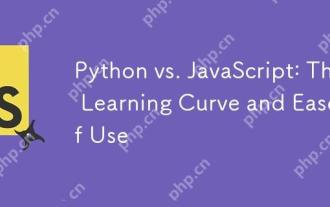
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
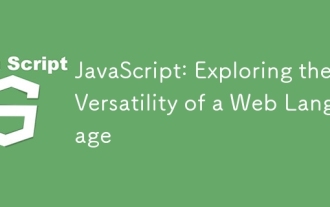
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
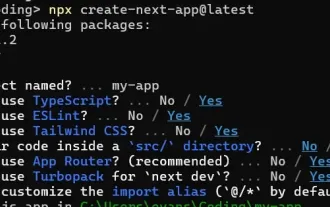
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
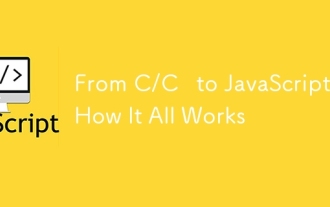
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
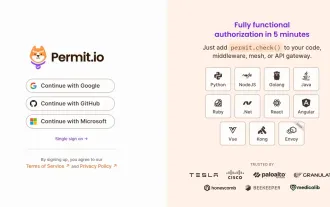
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
