Feature Flags with Express.js and GrowthBook
This guide walks through how to add GrowthBook feature flags to an Express.js application. It assumes you are starting from scratch, so if you already have an Express.js application, you can skip to step 2.
1. Create an Express.js app
First, install Express.js
npm install express
Then, create an index.js file with a simple hello world route
const express = require('express') const app = express() app.get('/', (req, res) => { res.send('Hello World!') }) // Listen on port 3000 const port = 3000 app.listen(port, () => { console.log(`Example app listening on port ${port}`) })
Finally, run the app with:
node index.js
Visit http://localhost:3000 and you should see a "Hello World!" response!
2. Create a GrowthBook Account
GrowthBook can be self-hosted, but for simplicity, we will use GrowthBook Cloud in this guide, which is free for simple projects like this.
Go to https://app.growthbook.io and create a new account if you don't have one yet.
Once you are logged in, create an SDK Connection and select Node.js as the language. This will generate a unique Client Key for you. Keep note of this key as we will use it in the next step.
3. Integrate the GrowthBook JavaScript SDK into the Express.js app
Create the file .env if it doesn't exist yet and add the generated key there:
GROWTHBOOK_API_HOST=https://cdn.growthbook.io GROWTHBOOK_CLIENT_KEY=
Now let's install the GrowthBook JavaScript SDK
npm install @growthbook/growthbook
Lets now modify the top of index.js file and create a GrowthBookClient instance:
const express = require('express') const app = express() const { GrowthBookClient } = require("@growthbook/growthbook") // GrowthBookClient instance const client = new GrowthBookClient({ apiHost: process.env.GROWTHBOOK_API_HOST, clientKey: process.env.GROWTHBOOK_CLIENT_KEY }); // Initialize it client.init().then((status) => { console.log("GrowthBook initialized", status); });
Lets also add a middleware to index.js that creates a user-scoped instance for every request. Make sure to place this above the route handlers:
app.use((req, res, next) => { // Attributes about the current user/request const userContext = { attributes: { // In a real app this would come from a cookie or session // We would also add more attributes like country, etc id: "123", } } // Make this available to all subsequent route handlers req.growthbook = client.createScopedInstance(userContext); next(); });
Lets re-start the Node process and make sure you see the "GrowthBook initialized" message in the console and the status is successful. Since we are using a .env file, we need to modify the command to tell Node.js to load it.
node --env-file=.env index.js
4. Create a Feature in GrowthBook
Back in the GrowthBook application, we can create a new feature. For this tutorial, we'll make a simple on/off feature flag that determines whether or not we show the message in Spanish.
The key we chose (spanish-greeting) is what we will reference when using the GrowthBook SDK.
We can now edit the route in index.js to use this flag:
app.get('/', (req, res) => { let message = "Hello World!"; if (req.growthbook.isOn("spanish-greeting")) { message = "Hola Mundo!"; } res.send(message); })
Now, if you restart the Node process and refresh the page in the browser, you will still see the original "Hello World!" because when we created the feature, we set it to be Off by default.
5. Target Specific Users
Now we can add rules to the feature to turn it on for specific users.
In the userContext we added, we hard-coded an id of "123". We can now use this id to create a rule in GrowthBook to turn on the feature for this user.
On the feature page, click the "Add Rule" button and select "Force Value" as the rule type. Then add targeting by attribute and save the rule. It should look something like this:
Rules start out in a draft state in GrowthBook. You need to publish the draft to make it live.
After publishing, restart the Node process once more and refresh the page. You should now see the message in Spanish. ¡Qué bueno!
Try changing the id in userContext to something else like 456, restart Node, and the response will now switch back to English.
Conclusion and Next Steps
In this tutorial, you learned how to use a simple feature flag in an Express app and target individual users. But this barely scratches the surface of what you can do with GrowthBook.
Here are a few next steps you can take:
- Use more advanced targeting
- Run A/B tests
View the full Node.js docs for more information on all of the options available in the GrowthBook SDK, including streaming updates, persistent caching, and more.
The above is the detailed content of Feature Flags with Express.js and GrowthBook. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










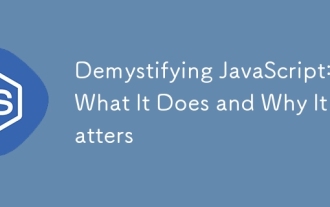
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
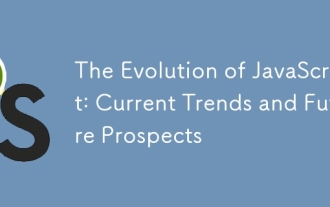
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
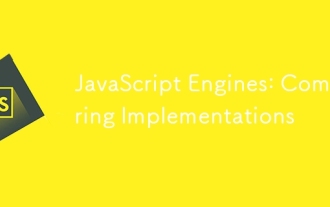
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
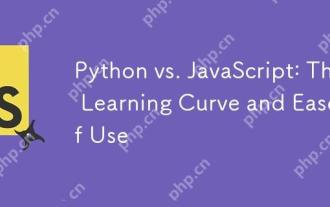
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
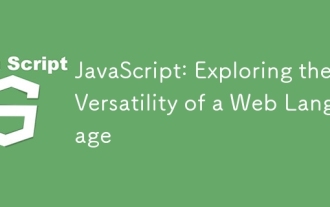
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
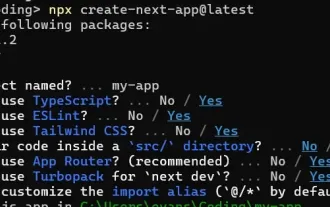
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
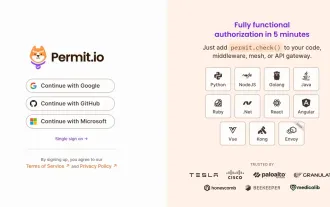
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
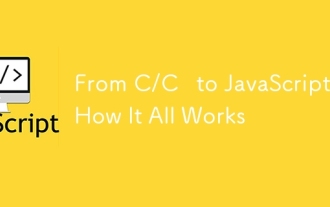
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
