


How Can I Efficiently Remove Non-Printable ASCII Characters (0-31 and 127) from a String?
Removing Non-Printable Characters from Strings
In situations where it's necessary to remove non-printable characters from strings, various approaches can be employed. This question focuses on eliminating characters ranging from 0-31 and 127.
Options for Removal:
preg_replace Regular Expression:
Using a regular expression with the preg_replace function is a versatile method that can tailor removal to specific ranges. For instance:
$string = preg_replace('/[\x00-\x1F\x7F-\xFF]/', '', $string);
This expression targets characters in the specified ranges and removes them from the string.
str_replace Character Replacement:
If the desired characters are limited, creating an array of them can avoid regular expressions. The str_replace function can then be used:
$badChars = [chr(0), chr(1), chr(2), ...]; $string = str_replace($badChars, '', $string);
Considerations:
Character Encoding:
The targeted ranges mentioned (0-31 and 127) align with ASCII's control characters. However, different character encodings may necessitate adjustments. For UTF-8, the '/u' modifier in the regular expression ensures proper matching.
Unicode Extension:
In UTF-8, additional non-printable characters beyond 0-31 and 127 can be present. To handle them, include the non-matching characters in the removal array or use the '/u' modifier with the regular expression.
Performance Benchmarking:
While regular expressions typically excel in efficiency, str_replace may perform better in certain scenarios. It's advisable to benchmark both approaches with the specific data being processed to determine the optimal solution.
The above is the detailed content of How Can I Efficiently Remove Non-Printable ASCII Characters (0-31 and 127) from a String?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


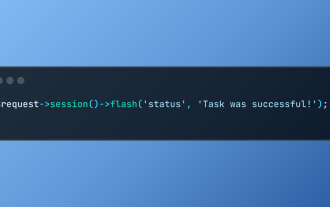
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
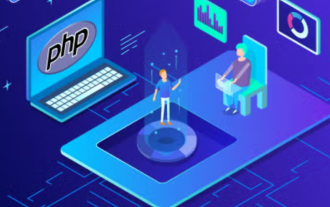
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
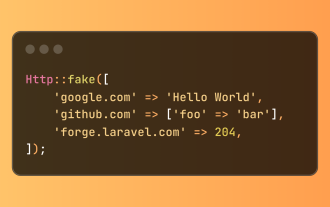
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
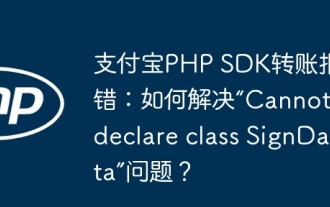
Alipay PHP...
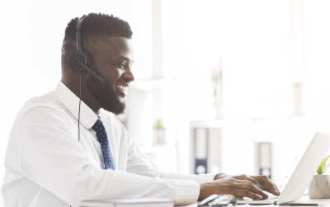
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
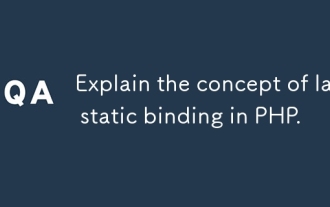
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
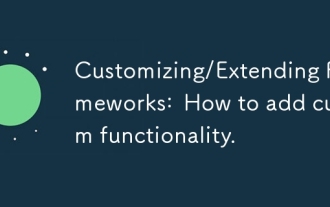
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
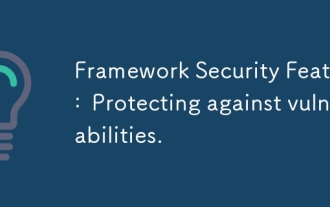
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
