


How Can I Effectively Handle Error Propagation in Multi-Layer Go Abstractions?
Error Propagation in Multi-Layer Abstractions
In Go, error handling across multiple levels of abstraction can become a challenge due to the necessity of continually passing the error upwards. This often leads to duplicate logging messages or the loss of contextual information.
Recommended Solution: Error Annotation
The preferred method of error handling is to annotate errors as they are passed through different abstraction levels. This allows for preserving the context of the original error while adding additional information at each level.
Using a library like "github.com/pkg/errors," the error handling can be implemented as follows:
// Wraps the original error with additional context. func (o *ObjectOne) CheckValue() error { if o.someValue == 0 { return errors.New("Object1 illegal state: value is 0") } return nil } // Annotates the original error with context. func (oT *ObjectTwoHigherLevel) CheckObjectOneIsReady() error { if err := oT.objectOne.CheckValue(); err != nil { return errors.Wrap(err, "Object2 illegal state: Object1 is invalid") } return nil } // Preserves the annotated error stack. func (oTh *ObjectThreeHiggerLevel) CheckObjectTwoIsReady() error { if err := oTh.ObjectTwoHigherLevel.CheckObjectOneIsReady(); err != nil { return errors.Wrap(err, "Object3 illegal state: Object2 is invalid") } return nil }
Usage:
o3 := &ObjectThreeHiggerLevel{} if err := o3.CheckObjectTwoIsReady(); err != nil { fmt.Println(err) }
Output:
Object3 illegal state: Object2 illegal state: Object1 illegal state: value is 0
This approach provides a clean and informative error handling mechanism that avoids duplicate logging and preserves contextual information.
Alternative: Error Extension
For simplicity, an alternative approach is to extend the original error with additional context using fmt.Errorf():
func (o *ObjectOne) CheckValue() error { if o.someValue == 0 { return fmt.Errorf("Object1 illegal state: value is %d", o.someValue) } return nil }
Usage:
o3 := &ObjectThreeHiggerLevel{} if err := o3.CheckObjectTwoIsReady(); err != nil { fmt.Println(err) }
Output:
Object3 illegal state: Object2 illegal state: Object1 illegal state: value is 0
This approach is less versatile than error annotation but is simpler to implement.
The above is the detailed content of How Can I Effectively Handle Error Propagation in Multi-Layer Go Abstractions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










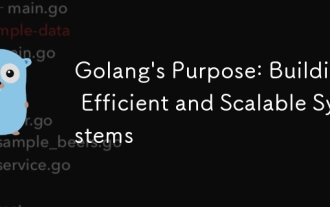
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
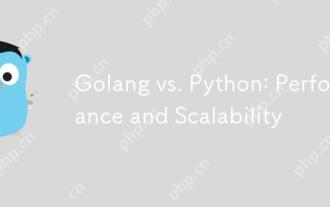
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
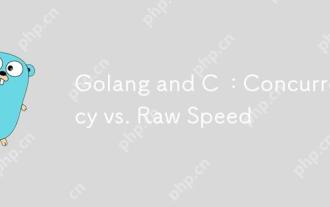
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
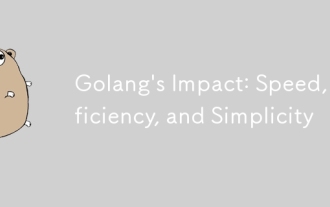
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
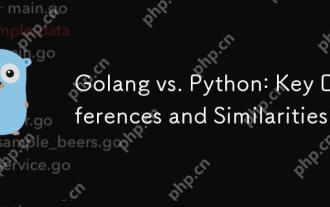
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
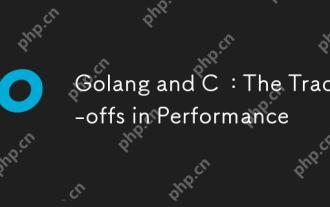
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
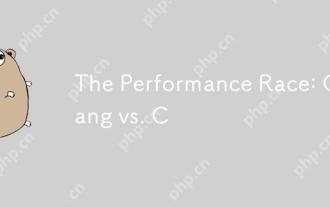
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
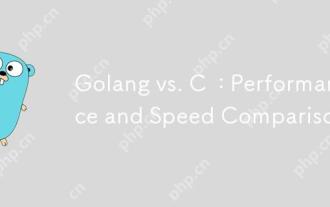
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
