How to Restrict JTextField Input to Positive Integers Only?
Restricting JTextField Input to Integers
To limit input to positive integers in a JTextField, utilizing a DocumentFilter is recommended over a KeyListener. A DocumentFilter provides a more comprehensive solution that handles various input scenarios.
DocumentFilter Implementation
A DocumentFilter can be implemented to validate input as it is inserted. This example filter, MyIntFilter, checks the entered text to ensure it represents a valid integer:
class MyIntFilter extends DocumentFilter { ... private boolean test(String text) { try { Integer.parseInt(text); return true; } catch (NumberFormatException e) { return false; } } ... }
This filter checks if the input text can be parsed as an integer. If valid, it allows the insertion. Otherwise, it prevents the insertion.
Applying the DocumentFilter
To apply the filter to your JTextField, use the setDocumentFilter method:
PlainDocument doc = (PlainDocument) textField.getDocument(); doc.setDocumentFilter(new MyIntFilter());
Advantages of using a DocumentFilter
- Handles various input scenarios, including pasting and cut/copy operations.
- Allows for complex input validation, such as checking for numeric limits or specific data formats.
- Simplifies code by keeping input validation in a separate class.
The above is the detailed content of How to Restrict JTextField Input to Positive Integers Only?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










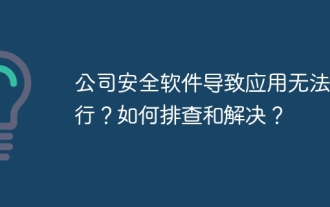
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
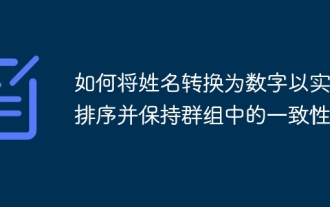
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
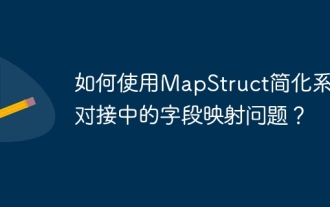
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
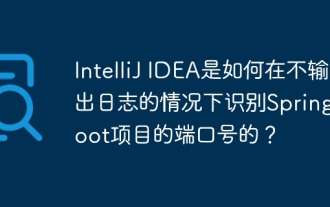
Start Spring using IntelliJIDEAUltimate version...
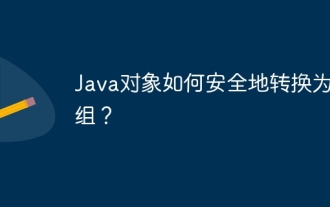
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
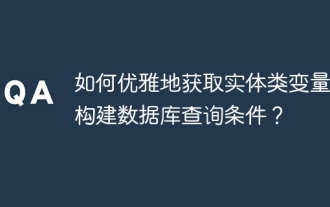
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
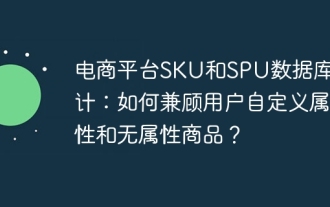
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
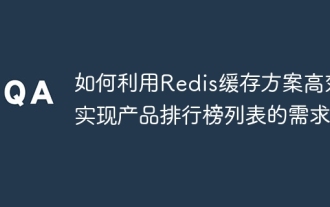
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
