


How Does the Tilde (~) Token Work in Go Generics to Define Underlying Types?
Understanding the Tilde Token (~) in Go Generics
In Go generics, the tilde token (~) plays a crucial role in defining the underlying type of a given type. It operates in the form ~T, indicating the collection of types that share T as their underlying type.
This concept is particularly useful in cases where you want to specify a constraint that allows for types that are derived from a specific underlying type. For instance, consider the following interface constraint:
1 2 3 |
|
In this example, the constraint defines an interface that can accept any type that is either an integer, a float, or a type whose underlying type is a string. This means that types like MyString, which defines a custom string type, can also satisfy this constraint as long as their underlying type remains string.
Underlying Types in Go
The term "underlying type" refers to the fundamental type that underlies a given type. In Go, this is determined based on the type declaration. For basic types like int, string, and bool, their underlying type is the type itself. However, for composite types like structs, slices, and interfaces, the underlying type is the type that is referenced in the type declaration.
Example Usage of the Tilde Token
The following code demonstrates the use of the tilde token:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
In this example, ExactSigned uses only exact types, which excludes MyInt8. On the other hand, constraints.Signed allows MyInt8 because it contains approximation elements like ~int8.
Note: Limitations of the Tilde Token
It's important to note that the tilde token cannot be used with type parameters. For example, the following code is invalid:
1 2 3 |
|
The above is the detailed content of How Does the Tilde (~) Token Work in Go Generics to Define Underlying Types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
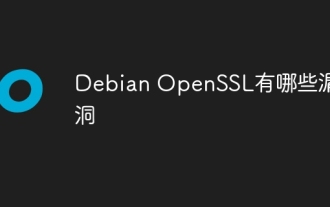
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
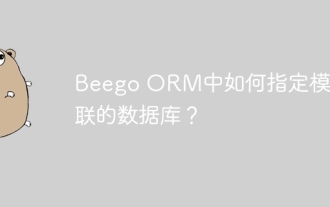
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
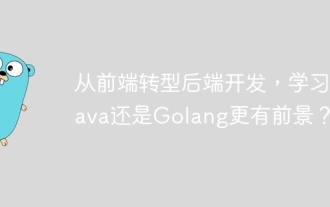
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
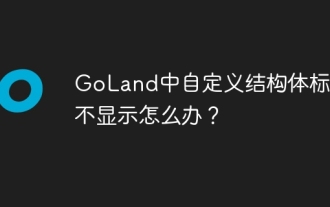
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
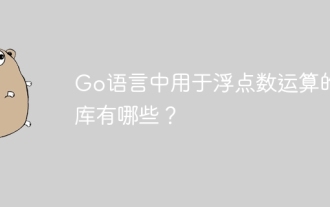
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
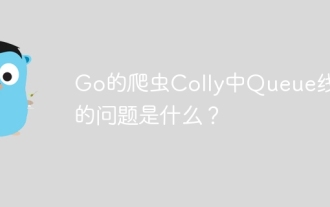
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
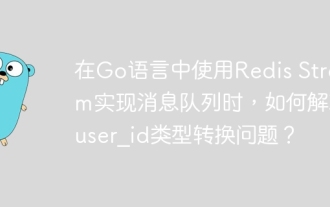
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
