Java Integer compareTo(): Why Comparison, Not Subtraction?
Java Integer compareTo(): Why Comparison Instead of Subtraction
The compareTo() method in Java's Integer class is designed to compare two Integer objects. Interestingly, it uses comparison operators instead of subtraction for this purpose. Let's explore the reason behind this choice.
Integer Overflow
When comparing two integers, using subtraction can lead to integer overflow, especially when dealing with large or negative values. This is because subtraction involves subtracting the second value from the first, which can produce a result that is outside the range of integers representable by the data type.
Consider the following example:
int thisVal = Integer.MAX_VALUE; int anotherVal = -1; int diff = thisVal - anotherVal; // Overflow occurs
In this case, the value of thisVal is the maximum value of an integer (2^31 - 1), and anotherVal is a negative value. Subtracting the latter from the former would produce a result that is larger than 2^31 - 1, causing an integer overflow and an incorrect comparison result.
Comparison Operators
Using comparison operators (e.g., <, >, ==) avoids the aforementioned overflow issue. These operators compare the bits of the two values directly, without performing any arithmetic operations. This ensures that the result will never overflow and will accurately represent the relationship between the operands.
Implementation
The implementation of compareTo() in Integer class takes this into account and uses the following logic:
public int compareTo(Integer anotherInteger) { int thisVal = this.value; int anotherVal = anotherInteger.value; return ( thisVal < anotherVal ? -1 : ( thisVal == anotherVal ? 0 : 1 ) ) ; }
This implementation uses the ternary conditional operator (?:) to determine the comparison result based on the values of thisVal and anotherVal. It ensures that the correct result is returned without causing integer overflow.
The above is the detailed content of Java Integer compareTo(): Why Comparison, Not Subtraction?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










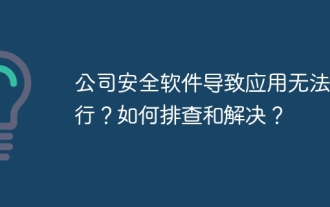
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
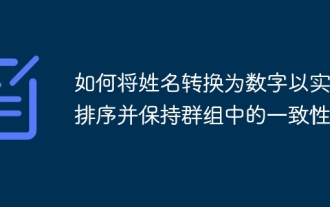
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
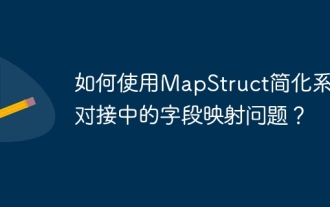
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
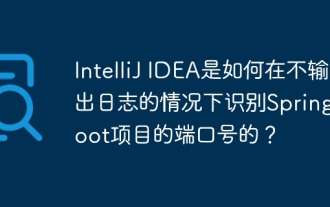
Start Spring using IntelliJIDEAUltimate version...
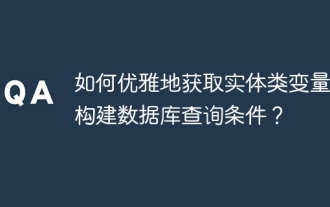
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
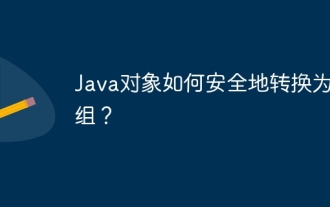
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
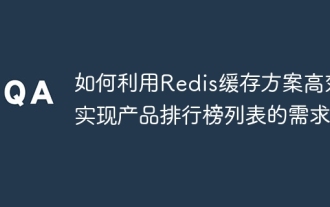
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
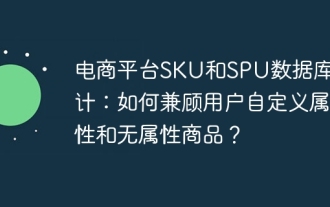
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
