


How to Redirect Different User Types to Their Respective Activities in Firebase?
Redirecting Multiple User Types to Their Respective Activities
In Firebase, managing different types of users and redirecting them appropriately is crucial for customizing their experience. To achieve this, it's essential to consider database schema and authorization listener adjustments.
Database Structure Considerations
When dealing with multiple user types, it's beneficial to incorporate user type information into the database. By creating a node for user types, you'll be able to differentiate between them. For instance, you could have a "users" node with subnodes for each user type (e.g., "STUDENTS", "TEACHERS", and "ADMINS"). Within each subnode, include essential user information, such as their UID, name, and type.
Modifying Authorization Listener for Multiple Users
Once the database structure is set up, modify the authorization listener to handle multiple user types. Here's a revised code example:
mAuthListener = new FirebaseAuth.AuthStateListener() { @Override public void onAuthStateChanged(FirebaseAuth firebaseAuth) { FirebaseUser firebaseUser = FirebaseAuth.getInstance().getCurrentUser(); if (mAuth.getCurrentUser() != null) { String uid = mAuth.getInstance().getCurrentUser().getUid(); DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference(); DatabaseReference uidRef = rootRef.child("users").child(uid); ValueEventListener valueEventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { if(dataSnapshot.child("Type").getValue(Long.class) == 1) { startActivity(new Intent(MainActivity.this, student.class)); } else if (dataSnapshot.child("TYPE").getValue(Long.class) == 2) { startActivity(new Intent(MainActivity.this, teacher.class)); } else if (dataSnapshot.child("TYPE").getValue(Long.class) == 3) { startActivity(new Intent(MainActivity.this, admin.class)); } } @Override public void onCancelled(@NonNull DatabaseError databaseError) { Log.d(TAG, databaseError.getMessage()); } }; uidRef.addListenerForSingleValueEvent(valueEventListener); } else{ Log.d("TAG", "firebaseUser is null"); } } };
Explanation:
In this code, we access the "users" node in Firebase and check the user's "Type" attribute within the onDataChange() method of the ValueEventListener. Based on the type (1, 2, or 3), we redirect the user to the appropriate activity (student, teacher, or admin).
This revised approach provides a flexible solution for handling multiple user types by leveraging database structure and authorization listener modifications.
The above is the detailed content of How to Redirect Different User Types to Their Respective Activities in Firebase?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










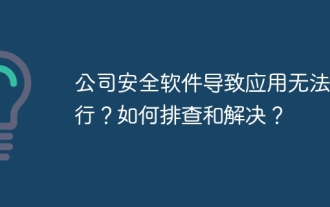
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
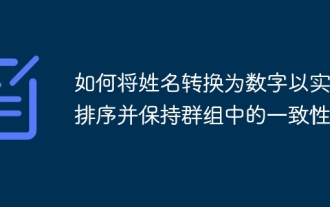
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
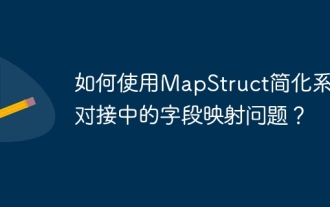
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
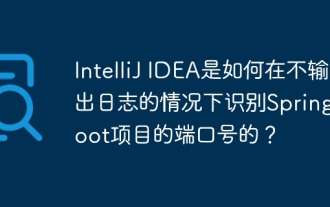
Start Spring using IntelliJIDEAUltimate version...
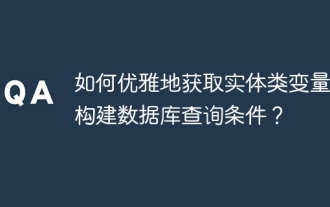
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
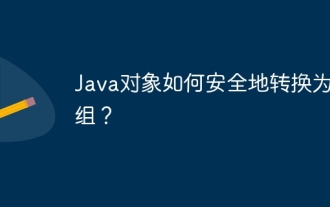
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
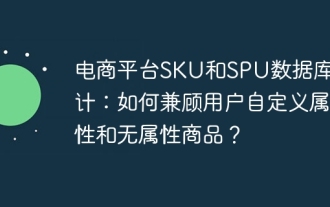
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
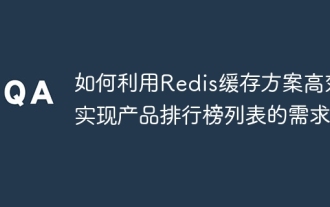
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
