


How Can a Custom Servlet Efficiently Serve Static Web Content Across Multiple Containers?
Serving Static Content with a Custom Servlet
In order to serve static content (e.g., images, CSS) for a web application deployed on multiple containers, a custom servlet can be utilized to ensure consistent URL handling.
Requirements for the Servlet
- Minimal external dependencies
- Efficient and reliable
- Compatibility with If-Modified-Since header (customizable getLastModified method)
- Optional support for gzip encoding and etags
Solution
While an alternative solution was suggested, it is possible to create a custom servlet that meets the stated requirements. Here's a potential implementation:
import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.File; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class StaticContentServlet extends HttpServlet { private Path filePath; @Override public void init() throws ServletException { super.init(); String filePathString = getServletConfig().getInitParameter("filePath"); if (filePathString == null) { throw new ServletException("Missing filePath init parameter"); } filePath = Paths.get(filePathString); } @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException { String path = request.getRequestURI().substring(request.getContextPath().length()); File file = filePath.resolve(path).toFile(); if (file.exists()) { response.setStatus(HttpServletResponse.SC_OK); response.setContentType(Files.probeContentType(file.toPath())); if (request.getDateHeader("If-Modified-Since") <= file.lastModified()) { response.setDateHeader("Last-Modified", file.lastModified()); } else { response.setStatus(HttpServletResponse.SC_NOT_MODIFIED); return; } Files.copy(file.toPath(), response.getOutputStream()); } else { response.sendError(HttpServletResponse.SC_NOT_FOUND); } } }
Usage
- In the web.xml deployment descriptor, map the servlet to the desired URL patterns. For example:
<servlet-mapping> <servlet-name>StaticContentServlet</servlet-name> <url-pattern>/static/*</url-pattern> </servlet-mapping>
- In the servlet's init() method, initialize the filePath instance variable to the location of the static content directory. This directory should contain the static files relative to the context root.
Benefits
This custom servlet provides reliable and customizable static content serving, meeting the specified requirements. It handles If-Modified-Since requests, allowing for conditional caching, and it can be configured to support other features (e.g., gzip encoding) via the servlet's init parameters.
The above is the detailed content of How Can a Custom Servlet Efficiently Serve Static Web Content Across Multiple Containers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










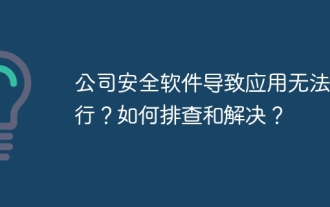
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
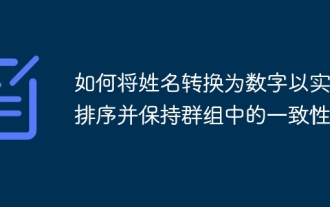
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
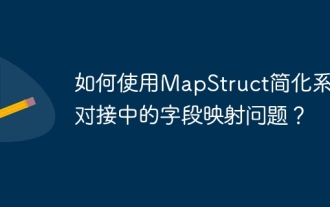
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
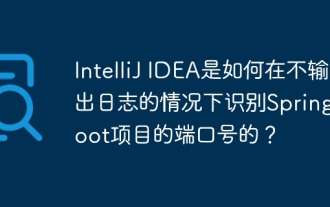
Start Spring using IntelliJIDEAUltimate version...
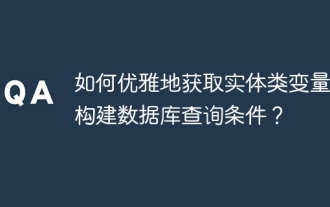
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
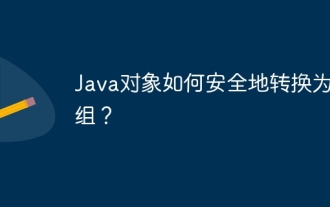
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
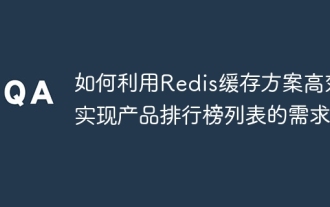
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
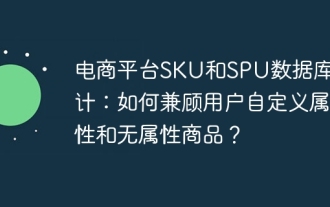
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
