How to Convert []byte to int in Go for TCP Communication?
Converting from []byte to int in Go for TCP Communication
Communication over TCP often requires exchanging data in the form of byte arrays ([]byte). However, in certain scenarios, you may need to convert these byte arrays to integers (int) for processing.
Conversion Methods
To convert a byte array to an integer in Go, you can use two primary methods:
1. Using encoding/binary:
The encoding/binary package provides functions that allow you to convert from various binary formats to Go types. Specifically, for converting byte arrays to integers, you can use:
binary.LittleEndian.Uint32(mySlice) // Converts to 32-bit unsigned int binary.BigEndian.Uint32(mySlice) // Converts to 32-bit unsigned int in big endian format
2. Custom Conversion:
You can also implement your conversion logic if the standard library functions don't meet your specific requirements. Here's an example of a custom conversion for converting a 4-byte array to an integer:
func bytesToInt(mySlice []byte) int { return int(mySlice[0]<<24 | mySlice[1]<<16 | mySlice[2]<<8 | mySlice[3]) }
Example Application:
In your client-server example, you can use the following code to send integers to the server:
package main import ( "encoding/binary" "net" ) func main() { // 2 numbers to send num1 := 100 num2 := 200 // Convert to byte arrays buf1 := make([]byte, 4) binary.LittleEndian.PutUint32(buf1, uint32(num1)) buf2 := make([]byte, 4) binary.LittleEndian.PutUint32(buf2, uint32(num2)) // Connect to server conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { // Handle error } // Send byte arrays to server if _, err := conn.Write(buf1); err != nil { // Handle error } if _, err := conn.Write(buf2); err != nil { // Handle error } }
The above is the detailed content of How to Convert []byte to int in Go for TCP Communication?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










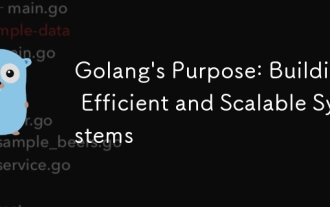
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
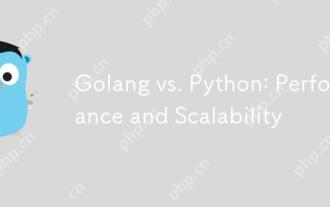
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
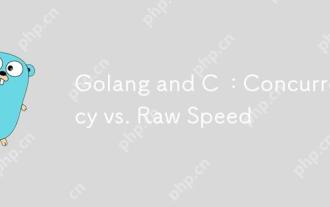
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
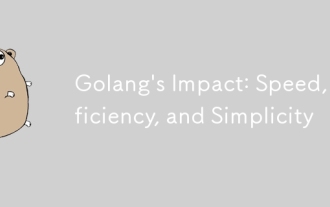
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
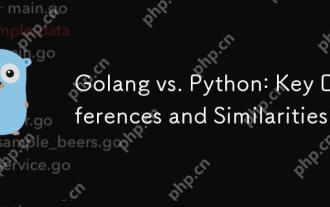
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
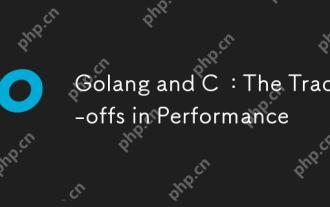
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
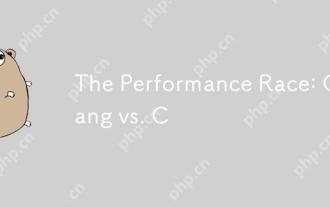
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
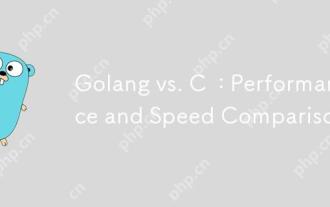
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
