


Why Do I Get an 'Undefined Reference to' Error with Template Class Constructors in C ?
"Undefined Reference to" Template Class Constructor
In C programming, template classes are often used to provide generic functionality that can be instantiated with different types. However, sometimes when instantiating a template class, you may encounter an "undefined reference to" error.
This error occurs when the compiler cannot find the implementation for the constructor of the template class. The compiler typically needs to see the implementation of the constructor in order to generate the code for the instantiated class.
Issue
In the provided code, the template class cola is defined in cola.h header file, but the implementation is in the cola.cpp file. When compiling the program, the compiler sees the template declaration in main.cpp and attempts to implicitly instantiate the cola class with float and string types.
However, since the compiler has not yet seen the implementation of the constructor in cola.cpp, it cannot generate the code for these instantiated classes, resulting in the "undefined reference to" error.
Solution
There are two approaches to resolve this issue:
Approach 1: Explicit Template Instantiation
Add explicit template instantiations at the end of the cola.cpp file:
template class cola<float>; template class cola<string>;
This explicitly instructs the compiler to generate code for the cola template class with the specified types.
Approach 2: Move Implementation to Header Files
Move the implementation of the template class constructor and member functions into the header file (cola.h and nodo_colaypila.h).
This ensures that the compiler sees the implementation when it instantiates the template class, eliminating the need for explicit instantiation.
Header File vs Translation Unit
In Approach 1, explicit instantiation is done at the end of the translation unit (a single compiled file). This means that all code in that file must be compiled before the instantiation.
In Approach 2, the implementation is in the header file, which is included in every translation unit that uses the template class. As a result, the implementation is available to the compiler regardless of the order of compilation.
Conclusion
Both approaches are valid solutions to the "undefined reference to" error with template classes. The choice depends on the specific needs of the project. Explicit instantiation is more flexible and allows for better control over which template specializations are generated. However, moving the implementation to header files is more common and provides more flexibility in using the template class.
The above is the detailed content of Why Do I Get an 'Undefined Reference to' Error with Template Class Constructors in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




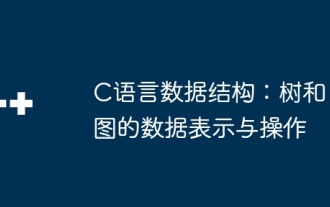
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
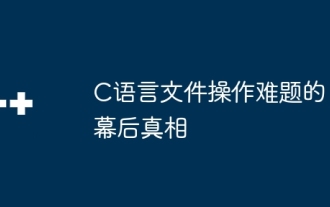
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
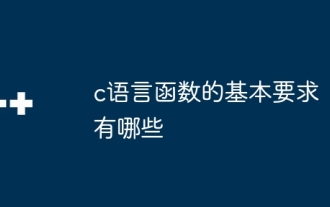
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
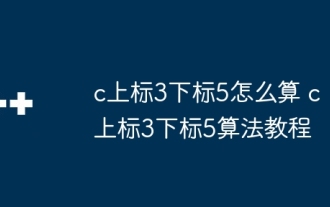
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
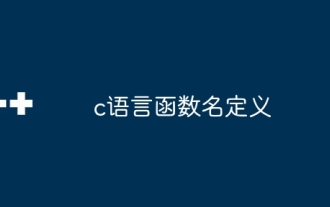
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
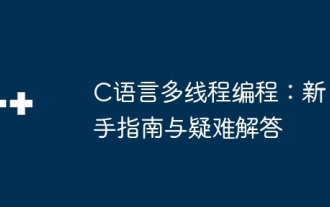
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
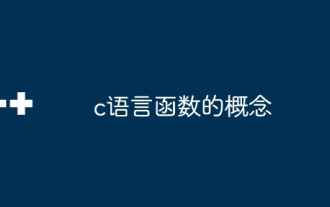
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
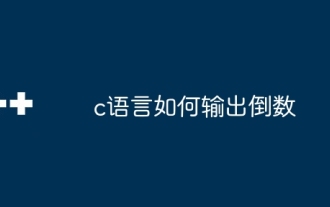
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
