


How Can I Efficiently Apply Multiple Functions to Multiple GroupBy Columns in Pandas?
Apply Multiple Functions to Multiple GroupBy Columns
Introduction
When working with grouped data, it is often necessary to apply multiple functions to multiple columns. The Pandas library provides several methods for achieving this, including the agg and apply methods. However, these methods have certain limitations and may not always meet specific use cases.
Using agg with a Dict
As mentioned in the question, it is possible to apply multiple functions to a groupby Series object using a dictionary:
grouped['D'].agg({'result1' : np.sum, 'result2' : np.mean})
This approach allows specifying the column names as keys and the corresponding functions as values. However, this only works for Series groupby objects. When applied to a groupby DataFrame, the dictionary keys are expected to be column names, not output column names.
Using agg with Lambda Functions
The question also explores using lambda functions within agg to perform operations based on other columns within the groupby object. This approach is suitable when your functions involve dependencies on other columns. While not explicitly supported by the agg method, it is possible to work around this limitation by manually specifying the column names as strings:
grouped.agg({'C_sum' : lambda x: x['C'].sum(), 'C_std': lambda x: x['C'].std(), 'D_sum' : lambda x: x['D'].sum()}, 'D_sumifC3': lambda x: x['D'][x['C'] == 3].sum(), ...)
This approach allows applying multiple functions to different columns, including those dependent on others. However, it can be verbose and requires careful handling of column names.
Using apply with a Custom Function
A more flexible approach is to use the apply method, which passes the entire group DataFrame to the provided function. This allows performing more complex operations and interactions between columns within the group:
def f(x): d = {} d['a_sum'] = x['a'].sum() d['a_max'] = x['a'].max() d['b_mean'] = x['b'].mean() d['c_d_prodsum'] = (x['c'] * x['d']).sum() return pd.Series(d, index=['a_sum', 'a_max', 'b_mean', 'c_d_prodsum']) df.groupby('group').apply(f)
By returning a Series with appropriately labeled columns, you can easily perform multiple calculations on the groupby DataFrame. This approach is more versatile and allows complex operations based on multiple columns.
Conclusion
Applying multiple functions to multiple grouped columns requires careful consideration of the data structure and the desired operations. The agg method is suitable for simple operations on Series objects, while the apply method offers greater flexibility when working with groupby DataFrames or performing complex calculations.
The above is the detailed content of How Can I Efficiently Apply Multiple Functions to Multiple GroupBy Columns in Pandas?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


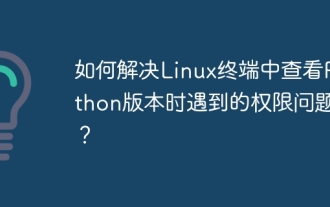
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
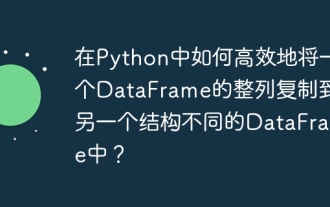
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
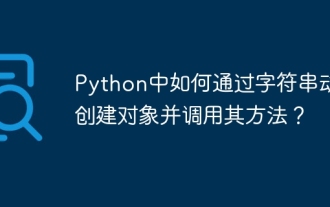
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
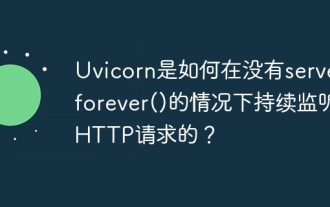
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
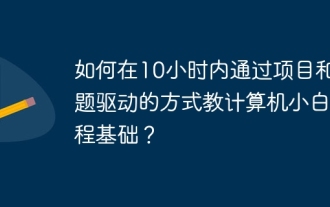
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
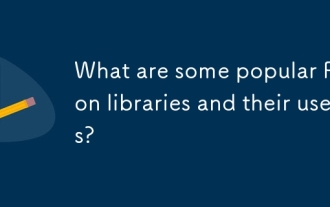
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
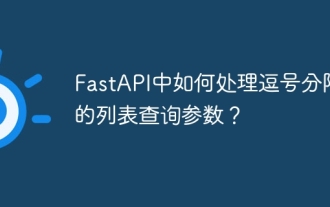
Fastapi ...
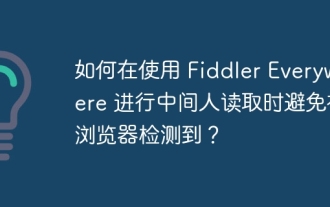
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
