


Why is my Canvas drawImage() failing to render images, and how can I resolve onload and CORS issues?
CanvasContext2D drawImage() conundrum: Tackling onload and CORS roadblocks
Problem Presentation:
Embarking on a canvas painting escapade, you find yourself faced with a baffling conundrum. Images rendered via drawImage() elude your grasp, leaving behind empty data URLs. Moreover, attempts to display the canvas yield no visible results, and the console remains eerily silent.
Unveiling the Perplexity:
The crux of the issue lies in the premature nature of your canvas artistry. Before drawImage() can wield its magic, the image must first complete its loading process. To appease this onLoad necessity, embrace the following strategy:
// Create the image var img = new Image(); // Define the onload function img.onload = function() { // Canvas setup var canvas = document.createElement("canvas"); canvas.width = img.width; canvas.height = img.height; var context = canvas.getContext("2d"); // Draw the image context.drawImage(img, 0, 0); // Obtain the data URL var dataURL = canvas.toDataURL(); // Perform desired actions with dataURL doSomething(dataURL); }; // Set the image source img.src = "http://somerandomWebsite/picture.png";
CORS Conundrum and Mitigation:
To ensure seamless toDataURL() and getImageData() execution, adhering to cross-origin resource sharing (CORS) is imperative. Prevent canvas 'tainting' by ensuring one of the following scenarios:
- Same-server image origin.
- External server with permissive CORS headers and img.crossOrigin set to "use-credentials".
- Anonymous CORS server with img.crossOrigin set to "anonymous".
Important Notes:
- CORS headers originate from the server, and the cross-origin attribute merely requests CORS usage.
- IE and Safari lack support for the cross-origin attribute.
Edge Case: Discrepancies in Image Sources:
If your images stem from a mix of same-server and CORS-compliant sources, consider utilizing the onerror event handler. Assign cross-origin as 'anonymous' to non-CORS servers and listen for errors.
function corsError() { this.crossOrigin = ""; this.src = ""; this.removeEventListener("error", corsError, false); } img.addEventListener("error", corsError, false);
The above is the detailed content of Why is my Canvas drawImage() failing to render images, and how can I resolve onload and CORS issues?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










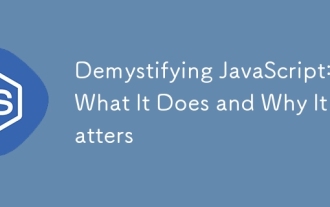
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
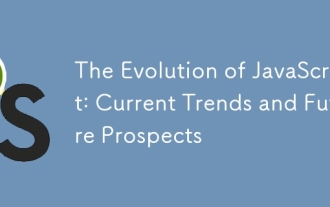
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
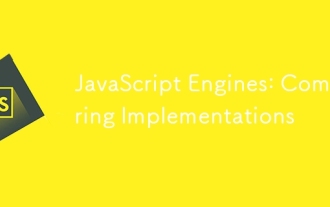
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
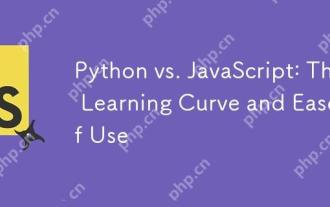
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
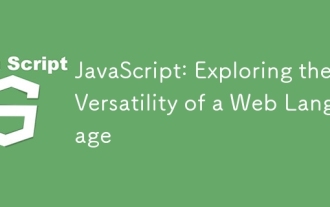
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
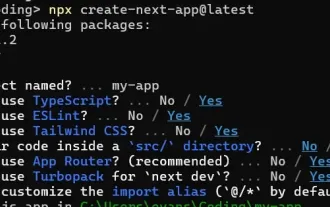
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
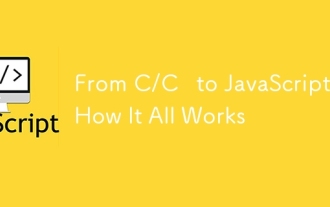
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
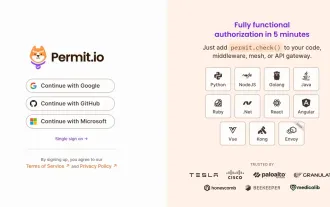
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
