


How Do I Prevent the Main Goroutine in a Go Program from Terminating Prematurely?
Main Goroutine Persistence in Go Programs
One may encounter the question of how to prevent the main goroutine of a Go program from terminating prematurely. In other words, the goal is to keep the program running indefinitely, except through explicit user intervention.
"Sleeping" the Main Goroutine
There are several approaches to make the main goroutine wait forever without consuming CPU resources:
-
An empty select statement:
select {}
Copy after login -
Receiving from an uninitialized channel:
<-make(chan int)
Copy after login -
Receiving from a nil channel:
<-(chan int)(nil)
Copy after login -
Sending to a nil channel:
(chan int)(nil) <- 0
Copy after login -
Locking a locked sync.Mutex:
mu := sync.Mutex{} mu.Lock() mu.Lock()
Copy after login
Quitting the Program
If a mechanism to terminate the program is desired, a simple channel can serve the purpose:
var quit = make(chan struct{}) func main() { // Startup code... // Blocking (waiting for quit signal): <-quit } // Quitting in another goroutine: close(quit)
Sleeping without Blocking
An alternative approach to keeping the program running without blocking the main goroutine is to use a long sleep duration:
time.Sleep(time.Duration(1<<63 - 1))
For extremely long-running programs, a loop of such sleeping statements can be employed:
for { time.Sleep(time.Duration(1<<63 - 1)) }
The above is the detailed content of How Do I Prevent the Main Goroutine in a Go Program from Terminating Prematurely?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










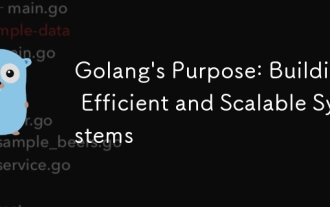
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
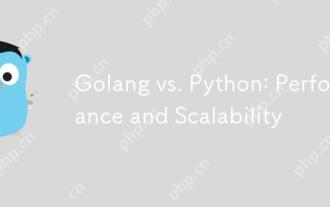
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
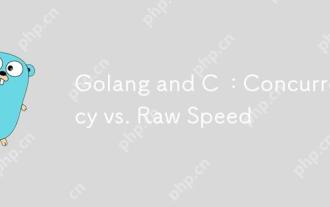
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
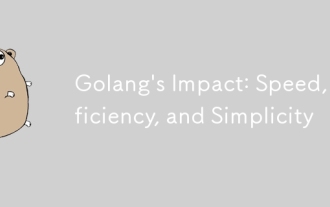
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
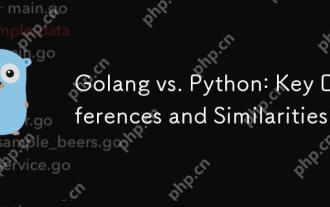
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
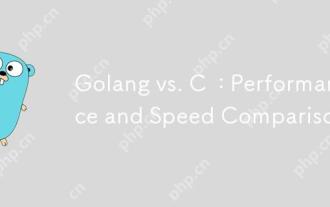
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
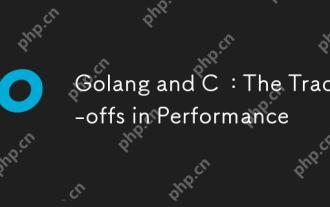
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
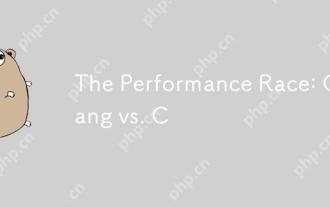
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
